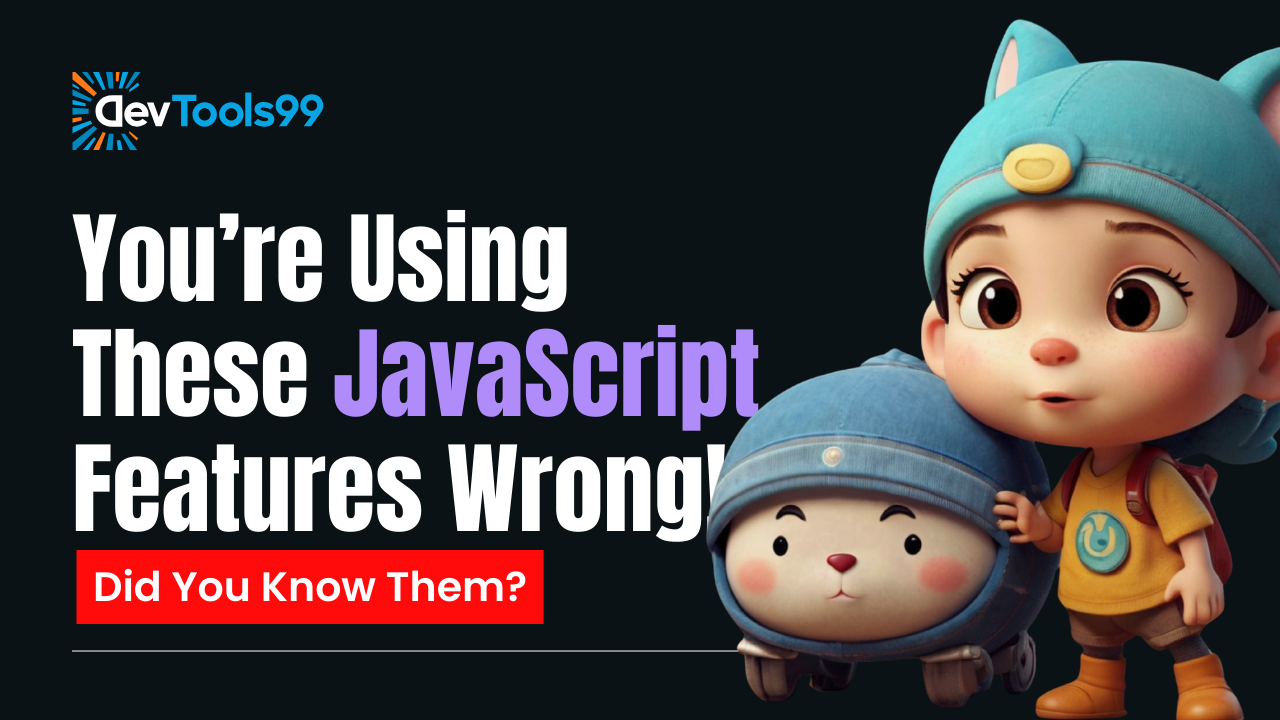
You’re Using These JavaScript Features Wrong!
JavaScript is a powerful language, but it's also full of potential pitfalls. Even experienced developers can sometimes misuse certain features, leading to bugs and confusing behavior. In this post, we'll go over some common JavaScript mistakes and how you can avoid them.
1. The Equality Trap: ==
vs ===
One of the most common mistakes in JavaScript is using the equality operator ==
when strict equality ===
should be used.
The ==
operator performs type conversion, meaning it tries to convert the operands to the same type before comparing them. This can lead to unexpected results. For example, 0 == '0'
returns true
, even though one is a number and the other is a string.
In contrast, the ===
operator enforces strict equality. It compares both the value and type, which helps avoid bugs caused by implicit type coercion. Using ===
ensures that no type conversion takes place, making your comparisons more predictable.
console.log(0 == '0'); // true
console.log(0 === '0'); // false
Tip: Always use ===
unless you have a specific reason to use ==
, which should be rare.
2. Misunderstanding this
in Arrow Functions
In JavaScript, the value of this
refers to the execution context of a function. Traditional functions bind their own this
, but arrow functions do not.
Arrow functions inherit the lexical scope of this
from their surrounding context. This makes them useful for callbacks or closures. However, this behavior can cause issues when using arrow functions as methods in an object because the value of this
may not refer to the object itself.
Here’s an example of how using arrow functions in the wrong context can cause problems:
const obj = {
name: 'JavaScript',
getName: () => {
console.log(this.name); // undefined, since arrow functions inherit outer scope's this
}
};
obj.getName();
To avoid this, stick to traditional function expressions when defining methods that rely on this
.
const obj = {
name: 'JavaScript',
getName: function() {
console.log(this.name); // JavaScript
}
};
obj.getName();
3. Overusing var
Instead of let
or const
Another common mistake is using var
to declare variables. The var
keyword is function-scoped, which means it does not respect block-level scope (e.g., within loops or if
statements). This can lead to unexpected behavior and make code harder to debug.
In contrast, let
and const
are block-scoped, meaning they are only accessible within the block in which they are defined.
const
: Use this for constants—values that should not change.let
: Use this for variables that can be reassigned.
if (true) {
var x = 5;
}
console.log(x); // 5 (x is still accessible outside the block)
if (true) {
let y = 10;
}
console.log(y); // ReferenceError: y is not defined
By sticking to let
and const
, you can write more predictable and error-free code.
4. Misusing for...in
with Arrays
The for...in
loop is meant for iterating over the enumerable properties of an object, not the elements of an array. When used with arrays, it can lead to unexpected results, as it iterates over both array indices and any additional properties that might have been added to the array’s prototype.
To loop over the elements of an array, you should use for...of
or a traditional for
loop:
const arr = [10, 20, 30];
for (let index in arr) {
console.log(index); // logs "0", "1", "2"
}
for (let value of arr) {
console.log(value); // logs "10", "20", "30"
}
Using for...of
ensures that you’re iterating over the actual values of the array rather than its properties.
5. Ignoring the Pitfalls of parseInt
Without a Radix
When using parseInt
to convert a string to an integer, you must always specify the radix (the base of the numeral system). Without it, parseInt
may interpret numbers starting with 0
as octal (base 8), leading to incorrect conversions.
For example, parseInt('010')
could return 8
instead of 10
.
To avoid this, always pass a radix of 10
for decimal numbers:
console.log(parseInt('010')); // 8
console.log(parseInt('010', 10)); // 10
Specifying the radix ensures that parseInt
behaves consistently and correctly, preventing surprises in your code.
Conclusion
These are just a few common JavaScript mistakes that can trip up even experienced developers. By understanding the behavior of ==
vs ===
, the difference between arrow functions and traditional functions, and when to use let
and const
over var
, you can write cleaner, more predictable code. Keep these tips in mind to avoid common pitfalls and improve your JavaScript coding practices.
Follow Us:
Stay updated with our latest tips and tutorials by subscribing to our YouTube Channel.