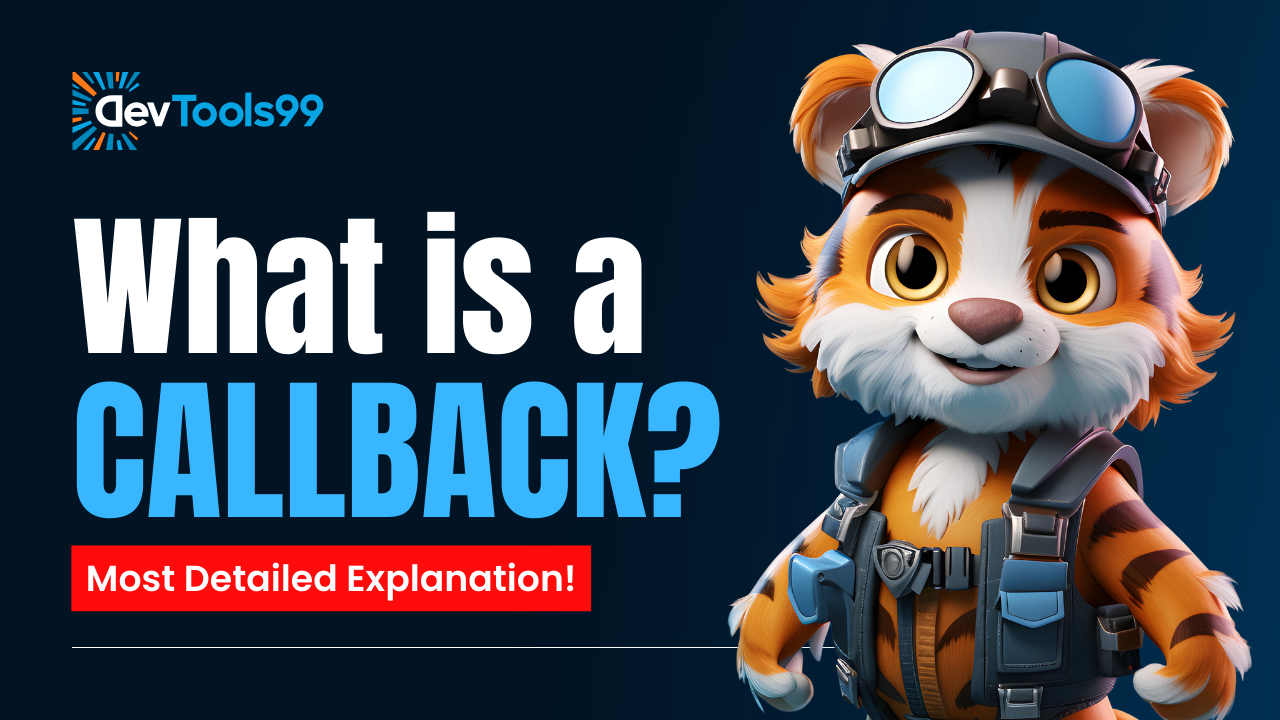
Understanding Callbacks in Programming with a Fun Party Analogy
Programming concepts can often seem abstract and tricky to grasp, but they become much clearer when explained through relatable analogies. Today, we’ll demystify the concept of callbacks in programming by comparing it to organizing a surprise birthday party. Imagine a scenario where you’re the party planner, and the entire event hinges on one critical factor: your friend arriving with the key to the venue. Let’s break it down step by step.
The Setup: Two Key Tasks
In our party analogy, you have two critical tasks to manage:
- Prepare the Venue: This involves decorating the venue, setting up tables, arranging seating, and ensuring everything is perfect for the surprise. From hanging streamers and setting up the sound system to organizing the snacks and beverages, every detail matters to create an unforgettable experience.
- Wait for the Key: This task depends entirely on your friend, who has the keys to the venue and is responsible for unlocking the doors at the right moment. Without the key, the venue remains inaccessible, and all your preparation efforts would be in vain.
While you might be eager to start decorating, there’s a problem: you can’t access the venue until your friend arrives with the key. Attempting to decorate without access would be ineffective and wasteful, much like trying to execute a function before its required data is available in programming.
The Challenge: Timing Your Efforts
This scenario reflects a common issue in programming: ensuring that tasks are performed in the correct order. In programming, if you try to execute a function before its necessary data or prerequisites are ready, you’ll likely encounter errors or unexpected behavior. Similarly, in our analogy, starting the decorations too early might result in misplaced items or incomplete setups, leading to a less-than-perfect surprise.
You could stand outside the venue, constantly checking if your friend has arrived, but this would waste time and energy. In programming, this is similar to polling, where a function repeatedly checks for a condition to be true before proceeding. Polling can be inefficient and resource-intensive, especially if the waiting period is long or unpredictable.
The Solution: Embracing Callbacks
Enter the concept of a callback. A callback is like an agreement or signal in our party analogy. Instead of continuously checking, you arrange with your friend to notify you as soon as they arrive with the key. This allows you to focus on other tasks in the meantime, such as preparing balloons, finalizing the guest list, or setting up the catering. Once the callback is triggered (your friend arriving with the key), you can immediately start decorating.
In programming, a callback works similarly. You define a function (the callback) and pass it as an argument to another function. The callback function is executed only when a specific condition is met or an event occurs. This ensures that your program proceeds smoothly without wasting resources.
Why Callbacks Are Efficient
Callbacks are powerful because they enable asynchronous behavior, which is essential for creating efficient and responsive programs. Here’s why they matter:
- Efficiency: By using callbacks, you avoid unnecessary waiting or constant checking. This keeps the system running smoothly and allows you to perform other tasks in parallel, much like how you can handle multiple party preparations without being stuck waiting for the key.
- Timing: Callbacks ensure that actions are executed only when the prerequisites are ready. This prevents errors and guarantees that the results are accurate and meaningful, similar to starting the decorations only when you have access to the venue.
- Flexibility: You can use callbacks in various scenarios, such as handling user interactions, processing data from APIs, or waiting for file uploads to complete. This versatility makes callbacks a fundamental tool in a programmer's toolkit.
Callbacks in Action: A Simple Code Example
Let’s translate our party analogy into code. Here’s a basic example of using a callback in JavaScript:
function getVenueKey(callback) {
console.log("Waiting for the key...");
setTimeout(() => {
console.log("Key is here!");
callback(); // Notify that the key has arrived
}, 3000); // Simulating a delay of 3 seconds
}
function decorateVenue() {
console.log("Decorating the venue...");
}
// Call the function and pass the callback
getVenueKey(decorateVenue);
In this example, the getVenueKey
function simulates waiting for the key. The setTimeout
function represents the delay in receiving the key, mimicking the unpredictability of real-life events. Once the key is ready, it triggers the decorateVenue
function, ensuring that decoration only starts at the right time.
Enhancing the Example: Multiple Callbacks and Error Handling
To further illustrate the power of callbacks, let’s expand our example to handle multiple tasks and potential errors:
function getVenueKey(callback, errorCallback) {
console.log("Waiting for the key...");
setTimeout(() => {
const success = true; // Change to false to simulate an error
if (success) {
console.log("Key is here!");
callback();
} else {
console.log("Failed to get the key.");
errorCallback("Unable to obtain the venue key.");
}
}, 3000);
}
function decorateVenue() {
console.log("Decorating the venue...");
}
function handleError(error) {
console.error("Error:", error);
}
// Call the function and pass the callbacks
getVenueKey(decorateVenue, handleError);
In this enhanced example, getVenueKey
accepts both a success callback and an error callback. This allows your program to handle both successful and unsuccessful attempts to obtain the key, providing a more robust and reliable flow. Similarly, in party planning, having a backup plan ensures that even if something goes wrong, you can adapt and still make the event a success.
Conclusion: The Beauty of Callbacks
Just as our party example demonstrates, callbacks are invaluable tools for managing tasks that depend on external factors or timing. They prevent wasted effort, ensure that actions are performed in the correct order, and make your code more efficient and elegant.
So, the next time you’re writing asynchronous code, think of it as planning a surprise party. With callbacks, you can ensure that every step happens at the perfect moment, creating a seamless and enjoyable experience for all involved.
Follow Us:
Stay updated with our latest tips and tutorials by subscribing to our YouTube Channel.