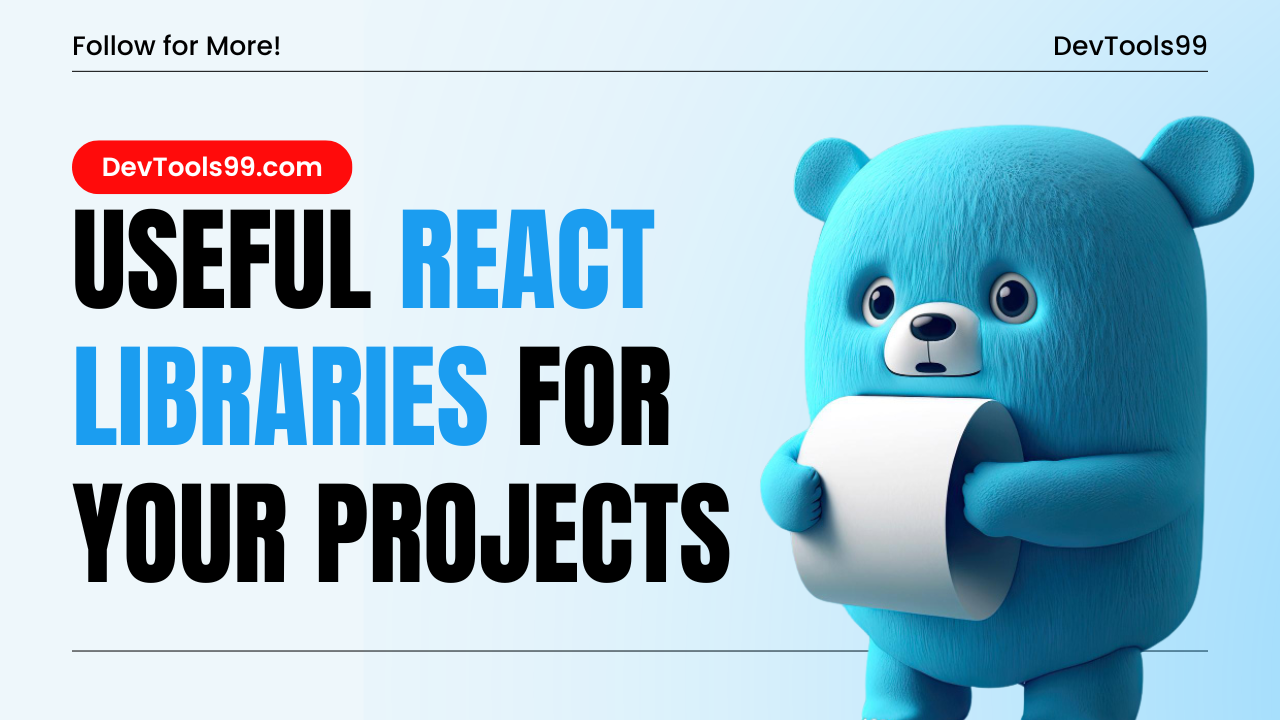
Useful React Libraries for Your Projects
React has a massive ecosystem with libraries that extend its functionality, making development easier and more efficient. From handling gestures to optimizing list rendering, these libraries offer specific tools that can improve your app's performance and user experience. Below are some useful React libraries to consider for your next project.
1. React-Konva
React-Konva is a powerful library that integrates HTML5 canvas with React applications. It allows you to create complex, interactive graphics and animations using a declarative syntax, much like React itself. With React-Konva, you can work with canvas elements more intuitively, abstracting much of the complexity involved in manipulating the canvas directly.
// Example usage of React-Konva
import { Stage, Layer, Rect } from 'react-konva';
function MyCanvas() {
return (
<Stage width={window.innerWidth} height={window.innerHeight}>
<Layer>
<Rect x={20} y={20} width={100} height={100} fill="red" />
</Layer>
</Stage>
);
}
Why Use It?
- Simplifies the use of canvas in React apps
- Enables the creation of dynamic, interactive graphics
- Great for building visualizations and custom animations
2. React-Gesture-Handler
React-Gesture-Handler is an essential library for adding touch and gesture support to your React applications. By leveraging the browser's native gesture handling, this library enables you to build applications with intuitive touch interactions, such as swiping, dragging, and pinching, making it perfect for mobile or touch-based interfaces.
// Example usage of React-Gesture-Handler
import { GestureHandlerRootView, PanGestureHandler } from 'react-native-gesture-handler';
function GestureExample() {
return (
<GestureHandlerRootView>
<PanGestureHandler onGestureEvent={onGestureEvent}>
<View style={{ width: 100, height: 100, backgroundColor: 'blue' }} />
</PanGestureHandler>
</GestureHandlerRootView>
);
}
Why Use It?
- Provides native-like touch gesture handling
- Supports smooth touch interactions
- Ideal for mobile and touch-based web apps
3. React-Flip-Move
React-Flip-Move is a library that makes it easy to animate the reordering of elements within lists or containers. When elements are added, removed, or rearranged, React-Flip-Move automatically animates the transition, making list updates more visually engaging and improving the overall user experience with fluid animations.
// Example usage of React-Flip-Move
import FlipMove from 'react-flip-move';
function AnimatedList({ items }) {
return (
<FlipMove>
{items.map(item => (
<div key={item.id}>{item.name}</div>
))}
</FlipMove>
);
}
Why Use It?
- Automatically animates changes in lists
- Makes UI more interactive and engaging
- Great for lists where elements frequently change
Learn more about React-Flip-Move
4. React-Virtualized
React-Virtualized is a performance-boosting library that optimizes the rendering of large lists and tabular data. It only renders the visible items on the screen, rather than rendering the entire list, which significantly reduces the memory and CPU load, especially when dealing with large datasets.
// Example usage of React-Virtualized
import { List } from 'react-virtualized';
function MyList({ items }) {
return (
<List
width={300}
height={600}
rowHeight={50}
rowCount={items.length}
rowRenderer={({ index, key, style }) => (
<div key={key} style={style}>{items[index]}</div>
)}
/>
);
}
Why Use It?
- Efficiently renders large lists and tables
- Improves app performance with lazy rendering
- Ideal for projects handling large datasets
Learn more about React-Virtualized
5. React-Toastify
React-Toastify simplifies the process of displaying toast notifications in your React applications. It provides a flexible and easy-to-use interface for adding customizable, attractive notifications that keep users informed about important events or updates without overwhelming the UI.
// Example usage of React-Toastify
import { ToastContainer, toast } from 'react-toastify';
import 'react-toastify/dist/ReactToastify.css';
function App() {
const notify = () => toast("Hello, world!");
return (
<div>
<button onClick={notify}>Show Toast</button>
<ToastContainer />
</div>
);
}
Why Use It?
- Provides customizable and stylish notifications
- Supports various types of messages (info, success, error)
- Non-intrusive way to keep users informed
Learn more about React-Toastify
6. React-Page-Transition
React-Page-Transition allows you to implement smooth and visually appealing transitions between different pages or components in your React app. This library enhances the navigation experience by providing fluid animations, giving your app a more polished and professional feel.
// Example usage of React-Page-Transition
import { PageTransition } from 'react-page-transition';
function MyComponent() {
return (
<PageTransition preset="moveToLeft" transitionKey="uniqueKey">
<div>New Page Content</div>
</PageTransition>
);
}
Why Use It?
- Adds visually appealing transitions to page changes
- Enhances navigation flow with smooth animations
- Improves the user experience for multi-page apps
7. React 360
React 360 is a library designed to build 360-degree image and video viewers in React. It simplifies the process of creating immersive, cross-platform 360-degree experiences, making it a valuable tool for developers looking to implement virtual reality (VR) or panoramic views in their apps.
// Example usage of React 360
import { ReactInstance } from 'react-360';
function init(bundle, parent, options) {
const vr = new ReactInstance(bundle, parent, {
...options,
});
vr.renderToSurface(
vr.createRoot('My360App', {}),
vr.getDefaultSurface()
);
}
Why Use It?
- Allows for the creation of 360-degree experiences
- Supports both image and video content
- Great for building immersive VR applications
Conclusion
These React libraries offer a wide range of functionalities, from touch gestures and animations to efficient rendering of large datasets. Depending on your project’s needs, integrating these tools can enhance performance, user experience, and overall development speed. Explore each one to see how they can make your React projects even more powerful.
Follow Us:
Stay updated with our latest tips and tutorials by subscribing to our YouTube Channel.