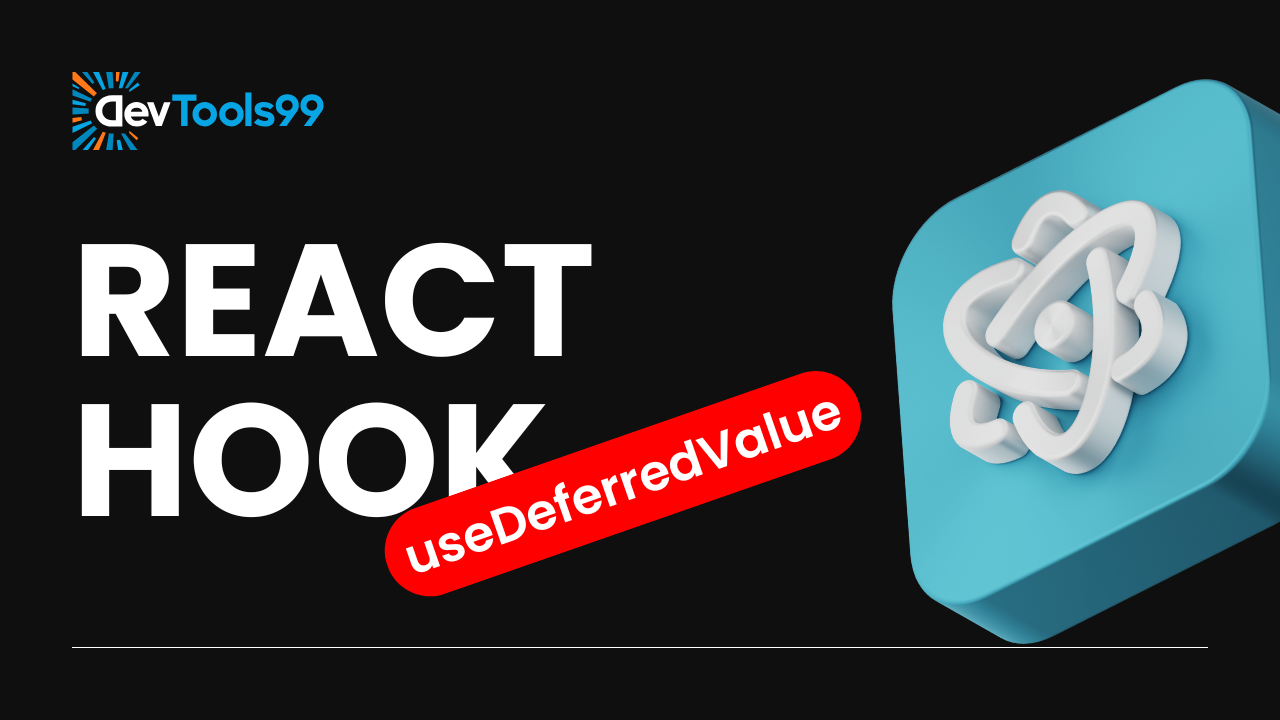
Mastering React Performance with useDeferredValue
React 18 introduced several features to optimize UI rendering, and useDeferredValue
is one of the most powerful additions. It provides developers with fine-grained control over state updates, ensuring smoother performance by deferring non-essential updates to a lower priority. But how does it work, and why should you care? Let’s explore!
What is useDeferredValue
?
At its core, useDeferredValue
allows you to delay the update of certain UI elements until higher-priority updates are complete. This is particularly beneficial in complex applications where certain calculations or rendering tasks can slow down the entire UI. By deferring these updates, React ensures the app remains responsive and interactive.
Unlike useTransition
, which is often used to mark entire state updates as low priority, useDeferredValue
focuses on individual values. This makes it ideal for optimizing specific components or parts of a user interface without affecting the whole app.
Code Example: Using useDeferredValue
in React
Below is an example of how to use useDeferredValue
to optimize a search feature. The code demonstrates how the hook helps keep the input responsive while handling a computationally expensive filtering operation.
import React, { useState, useDeferredValue } from 'react';
function DeferredExample({ items }) {
const [searchQuery, setSearchQuery] = useState('');
const deferredQuery = useDeferredValue(searchQuery);
const filteredItems = items.filter(item => item.includes(deferredQuery));
return (
<div>
<input
type="text"
value={searchQuery}
onChange={(e) => setSearchQuery(e.target.value)}
placeholder="Search items..."
/>
<ul>
{filteredItems.map((item, index) => (
<li key={index}>{item}</li>
))}
</ul>
</div>
);
}
In this example, the useDeferredValue
hook ensures that the searchQuery
input updates in real-time while the potentially expensive filtering operation is deferred to a lower priority.
Real-Life Use Cases
The potential applications of useDeferredValue
are vast. Here are some practical scenarios where it can shine:
- Dynamic Tables: Imagine a table displaying thousands of rows with complex calculations, like tax computations or real-time updates. With
useDeferredValue
, you can render the basic table structure first and defer the complex calculations for a smoother user experience. - Search and Filter Operations: Filtering large datasets in response to user input can cause noticeable lag. By deferring updates,
useDeferredValue
keeps the search box responsive while the filtering logic catches up in the background. - Interactive Visualizations: In data-heavy dashboards, rendering charts or graphs can be expensive. Use
useDeferredValue
to delay rendering non-critical elements without blocking interactive features. - Lazy-Loaded Content: When implementing infinite scrolling, defer updates to off-screen elements while prioritizing the currently visible content.
How It Improves User Experience
A key benefit of useDeferredValue
is how it improves perceived performance. By prioritizing critical updates, it reduces UI "jank"—the delays or freezes users experience during intensive computations. For example:
- Input fields remain responsive even if the associated logic is heavy.
- Visual components, like tables or charts, load incrementally without overwhelming the main thread.
- Applications handle resource-intensive tasks like filtering or rendering complex UIs without losing interactivity.
Best Practices and Pitfalls
While useDeferredValue
is powerful, it’s not a silver bullet. Consider these tips to get the most out of it:
- Use selectively: Only defer updates that aren't critical to the immediate user experience.
- Combine with memoization: Use
useMemo
oruseCallback
to avoid redundant computations for deferred values. - Test for regressions: Ensure deferred updates don't cause unexpected delays in critical parts of the UI.
Conclusion
The useDeferredValue
hook is a game-changer for building performant React applications. By deferring non-critical updates, it ensures smoother interactions, responsive UIs, and an overall improved user experience. Whether you're optimizing a dynamic table, a search bar, or a complex visualization, useDeferredValue
is a valuable tool in your React arsenal. Start using it today to make your applications not only functional but also delightfully fast!
Follow Us:
Stay updated with our latest tips and tutorials by subscribing to our YouTube Channel.