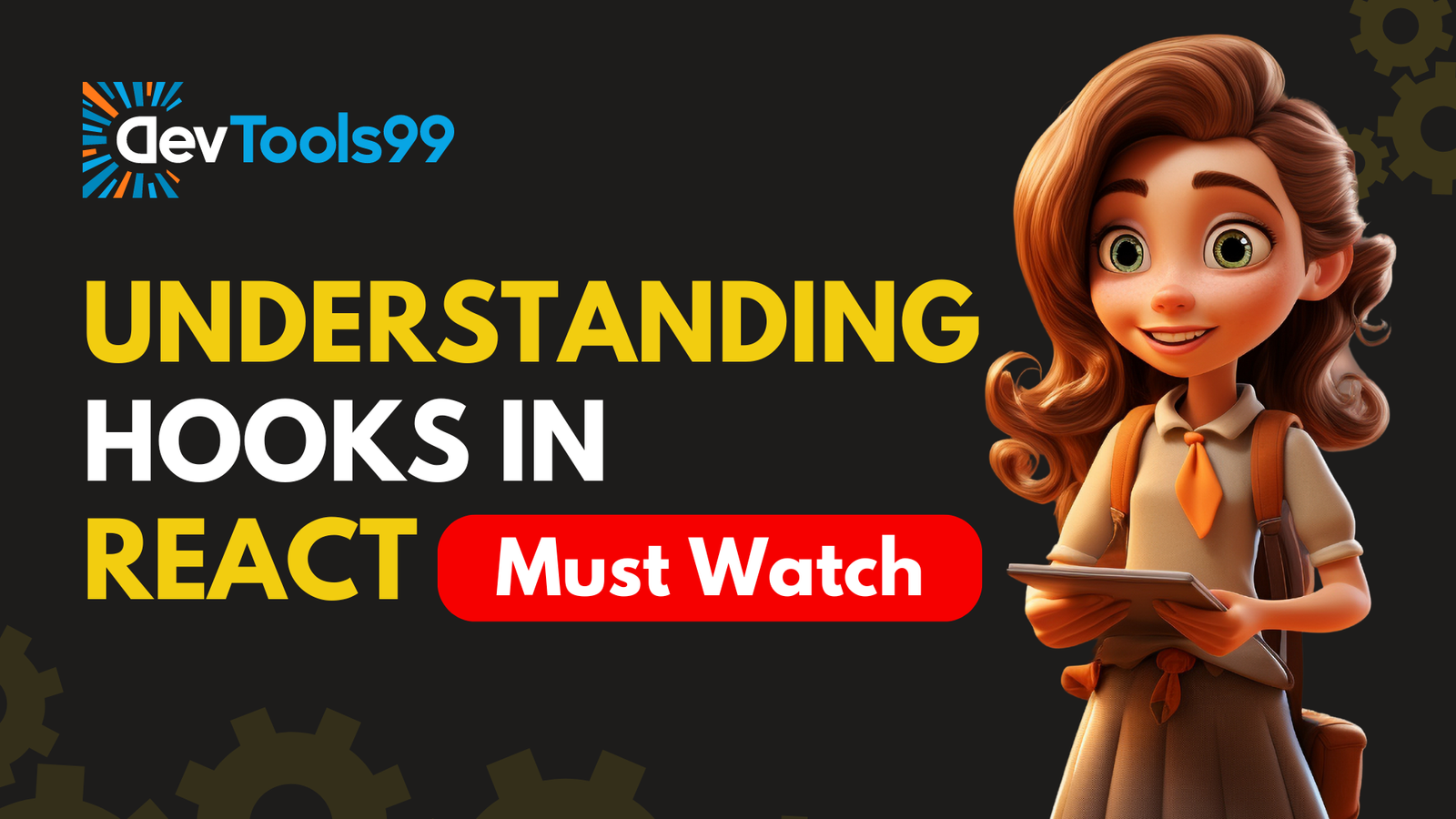
Understanding Hooks in React.
React hooks are a way to manage state and side effects in functional components in React. They make it easier to add state and functionality to your components without needing to convert them into class components.
1. useState:
This hook allows you to add state to your functional components. it takes an initial value and returns an array with two elements: the current and a function to update that state.
2. useEffect:
This hook is used for handeling side effects, like data fetching, DOM manipulation, and more. It takes two arguement: a function that contains your side effect code, and an array of dependencies that specify when the effect should run. if no dependency is specified, the side effect code runs only once when it is mounted initially.
3. useContext:
The useContext hook allow you to access a context (a global data store that you can uses to share information across various parts of your application without the need to pass props through every level of the component tree). You pass a context object created using React.createContext and receive the current context value.
4. useReducer:
This hook is an alternative to useState when you have complex state logic. It takes a reducer function and an initial state or data, and returns the current state and a dispatch function to update it.
5. useMemo:
useMemo helps optimize your application by memoizing(caching) the result of a function. It's particularly useful for preventing unnecessary recalculation of values, which can improve performance in your app.
6. useCallback:
While useMemo memoizes values, useCallback helps you optimize your application by memoizing()caching function. It's especially useful for preventing unnecessary function recreations, which can improve performance.
By using useCallback, you ensure that the handleClick function is only recreated if the dependencies change.
7. custom hooks:
You can create your custom hooks to share stateful logic between components. They typically start with "use" and can encapsulate any hook, or combinations of hooks, to provide a specific behaviour or functionality.
Like, Share and Subscribe #DevTools99 for more useful videos, tools info and #tutorials . Thank you!