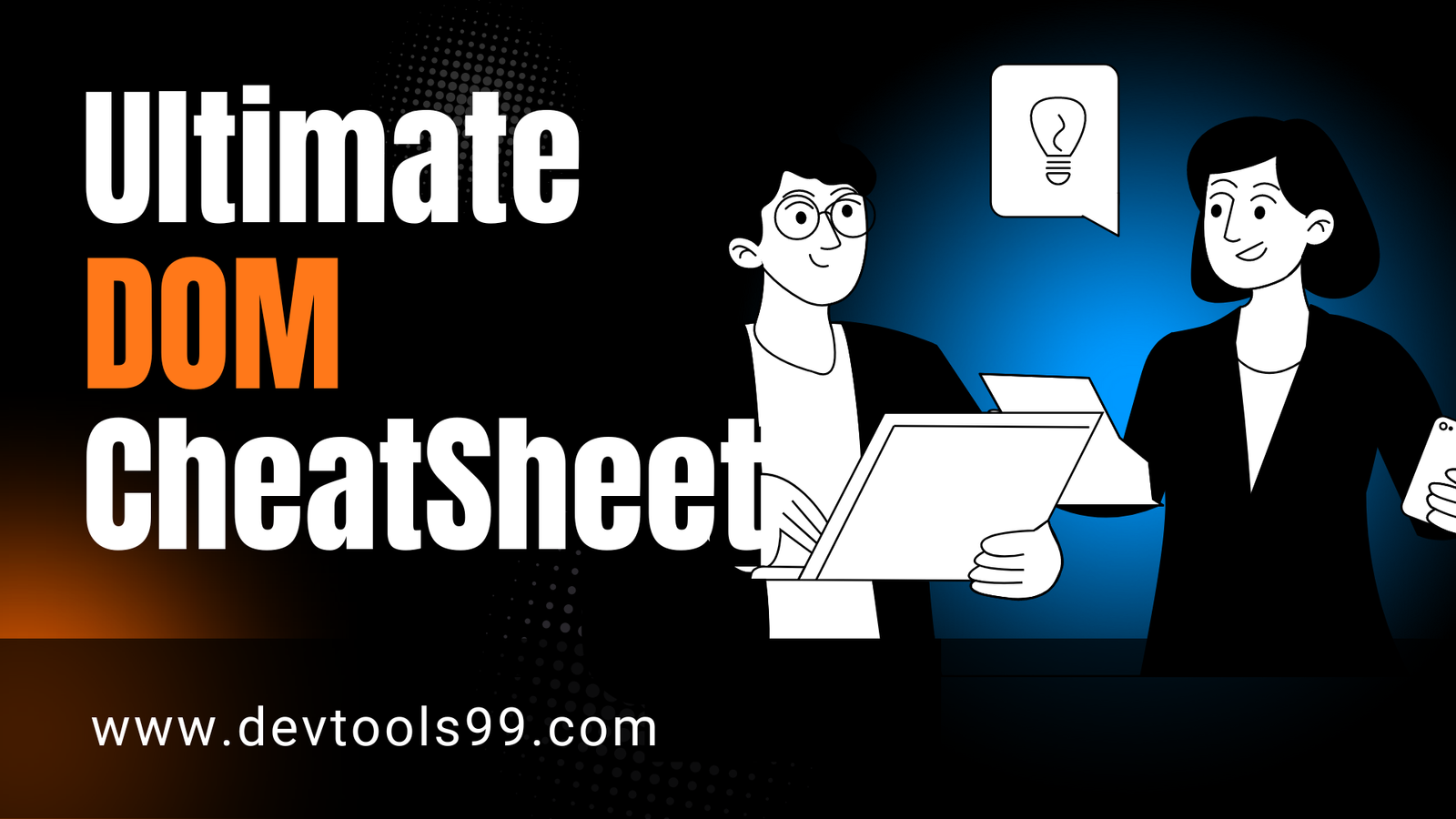
Ultimate DOM Cheat Sheet
Accessing Elements
In order to update a page, we need to find or select those elements that we want to update. Here are some methods:
getElementById(id)
:Selects an individual element within a document using a specific id. This method returns the element that has the ID attribute with the specified value.
Example:
document.getElementById("header");
querySelector(selector)
:Uses a CSS selector to select the first matching element within a document. This method returns the first element that matches the specified selector.
Example:
document.querySelector(".main-content");
getElementsByClassName(className)
:Allows you to select all elements with a given class attribute. This method returns a live HTMLCollection of elements with the specified class name.
Example:
document.getElementsByClassName("item");
getElementsByTagName(tagName)
:Locates all elements that match a given tag name. This method returns a live HTMLCollection of elements with the specified tag name.
Example:
document.getElementsByTagName("p");
querySelectorAll(selector)
:Uses a CSS selector to select one or more elements. This method returns a static NodeList of all elements that match the specified selector.
Example:
document.querySelectorAll("div > p");
Traversing the DOM
The process of selecting another element based on its relationship to a previously selected element:
parentNode
:Locates the parent element of an initial selection. This property returns the parent node of the specified node.
Example:
element.parentNode;
previousSibling
:Finds the previous sibling of a selected element. This property returns the previous sibling node of the specified node.
Example:
element.previousSibling;
nextSibling
:Finds the next sibling of a selected element. This property returns the next sibling node of the specified node.
Example:
element.nextSibling;
firstChild
:Finds the first child of a selected element. This property returns the first child node of the specified node.
Example:
element.firstChild;
Accessing & Updating Content
The innerHTML
and textContent
properties can be used to access or update content:
innerHTML
:Get or set the HTML content of an element. This property returns the HTML content inside an element as a string.
Example:
element.innerHTML = "<p>Hello, World!</p>";
textContent
:Get or set the text content of an element. This property returns the text content inside an element as a string.
Example:
element.textContent = "Hello, World!";
Modify Element
style
:Used to modify the style of the element. The
style
property returns the inline styles of an element and allows you to set CSS properties directly on an element.Example:
button.style.color = "#000000";
className
:Change the value of the class attribute for an element. This property gets and sets the value of the class attribute of the specified element.
Example:
element.className = "new-class";
setAttribute(name, value)
:Sets an attribute of an element. This method adds the specified attribute to an element and gives it the specified value.
Example:
element.setAttribute("data-id", "12345");
removeAttribute(name)
:Removes an attribute from an element. This method removes the specified attribute from an element.
Example:
element.removeAttribute("data-id");
Modify Element Class
classList.add(className)
:Adds a class to the element. This method adds the specified class value to the element if it is not already present.
Example:
button.classList.add("cn");
classList.remove(className)
:Removes a class from the element. This method removes the specified class value from the element if it is present.
Example:
button.classList.remove("cn");
classList.toggle(className)
:Toggles a class on the element. This method adds the specified class value to the element if it is not present and removes it if it is present.
Example:
button.classList.toggle("cn");
classList.contains(className)
:Checks if the element contains a class. This method returns
true
if the element contains the specified class value andfalse
otherwise.Example:
button.classList.contains("cn");
classList.replace(oldClass, newClass)
:Replaces an existing class with a new one. This method removes the specified old class value and adds the specified new class value to the element.
Example:
button.classList.replace("old", "new");
Adding Content
To add new elements to the page, there's 3 steps:
- Create a new list item and store it in a variable.
var newListItem = document.createElement("li");
- Update the text content of the list item.
newListItem.textContent = "Popcorn";
- Add the list item as a child of the
<ul>
.document.querySelector("ul").appendChild(newListItem);
Events
There are a load of events that can trigger a function. Here's a few of them:
click
:When a button is pressed and released on a single element. This event is triggered when an element is clicked.
Example:
button.addEventListener("click", function() { alert("Button clicked!"); });
keydown
:When the user first presses a key on the keyboard. This event is triggered when a key is pressed down.
Example:
document.addEventListener("keydown", function(event) { console.log("Key pressed:", event.key); });
keyup
:When the user releases a key on the keyboard. This event is triggered when a key is released.
Example:
document.addEventListener("keyup", function(event) { console.log("Key released:", event.key); });
focus
:When the element has received focus. This event is triggered when an element gains focus.
Example:
input.addEventListener("focus", function() { console.log("Input focused!"); });
blur
:When an element loses focus. This event is triggered when an element loses focus.
Example:
input.addEventListener("blur", function() { console.log("Input lost focus!"); });
submit
:When the user submits a form. This event is triggered when a form is submitted.
Example:
form.addEventListener("submit", function(event) { event.preventDefault(); console.log("Form submitted!"); });
load
:When the page has finished loading. This event is triggered when the entire page (including all dependent resources such as stylesheets and images) has loaded.
Example:
window.addEventListener("load", function() { console.log("Page fully loaded!"); });
resize
:When the browser window has been resized. This event is triggered when the browser window is resized.
Example:
window.addEventListener("resize", function() { console.log("Window resized!"); });
scroll
:When the user scrolls up or down on the page. This event is triggered when an element's scrollbar is being scrolled.
Example:
window.addEventListener("scroll", function() { console.log("Page scrolled!"); });
Follow Us:
Stay updated with our latest tips and tutorials by subscribing to our YouTube Channel.