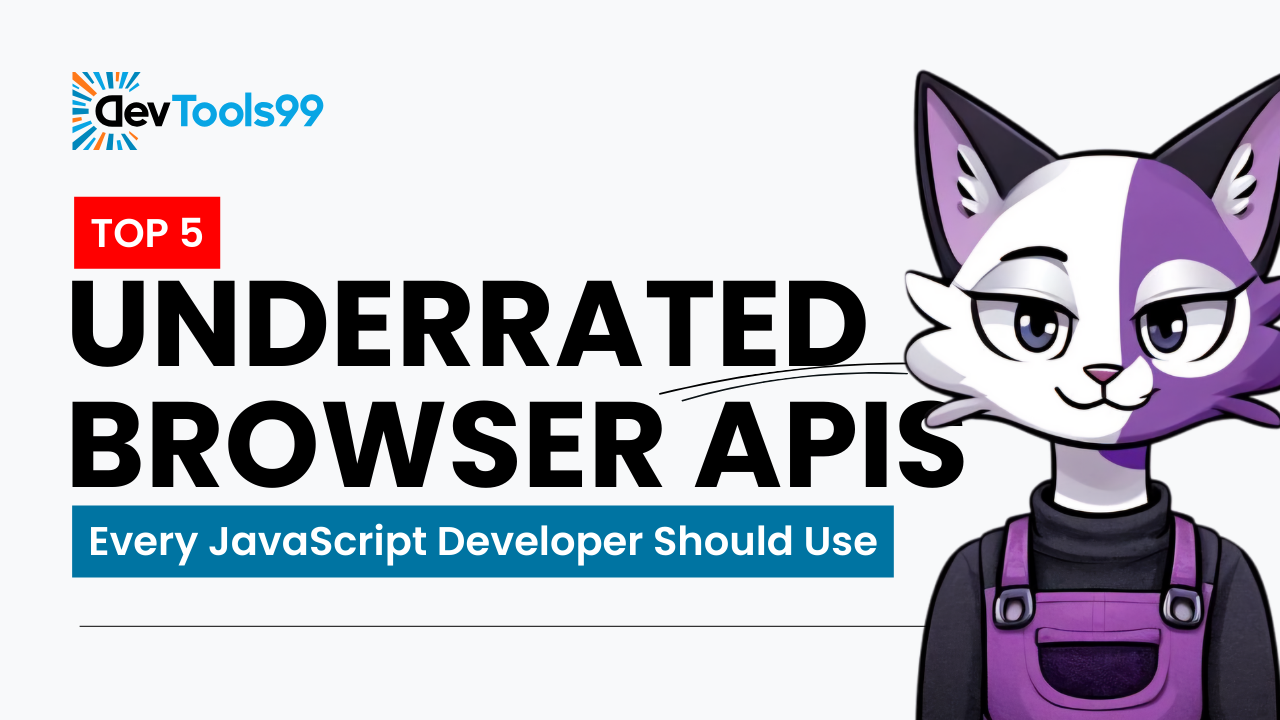
Top 5 Underrated Browser APIs Every JavaScript Developer Should Use
JavaScript is an incredibly versatile language, powering a vast array of interactive web applications. While most developers are familiar with popular browser APIs such as DOM manipulation or the Fetch API, there’s a treasure trove of lesser-known APIs that can elevate your applications to new heights. These hidden gems offer unique functionalities to build smarter, faster, and more user-friendly web experiences.
In this guide, we’ll explore five underrated browser APIs, delving into their potential and demonstrating how they can enrich your JavaScript skills and projects, enhancing your web development toolkit.
1. Clipboard API
The Clipboard API is a game-changer for creating seamless copy-paste interactions within web applications. It allows developers to programmatically copy and paste content—such as text, URLs, or even images—directly to and from the user’s clipboard. This is particularly useful for applications that involve a lot of content interaction, like document editors or collaboration tools.
A common use case is implementing a "Copy to Clipboard" button, perfect for sharing code snippets, promotional links, or any reusable data, enhancing user interaction and interface efficiency.
document.querySelector('#copyBtn').addEventListener('click', async () => {
try {
await navigator.clipboard.writeText('Copied Text!');
console.log('Text successfully copied to clipboard');
} catch (err) {
console.error('Failed to copy text: ', err);
}
});
By simplifying the content sharing process, the Clipboard API not only enhances user convenience but also significantly improves workflows in web applications.
2. Intersection Observer API
The Intersection Observer API provides a powerful option for managing and controlling element visibility within the browser’s viewport. This API is invaluable for efficiently handling events like lazy-loading images, implementing infinite scrolling, or activating animations just as they enter a user’s view, which significantly improves the performance of web applications.
It eliminates the need for complex and resource-intensive JavaScript scroll handlers, by allowing developers to asynchronously monitor changes in the intersection of a target element with an ancestor element or with a top-level document's viewport.
const observer = new IntersectionObserver((entries) => {
entries.forEach(entry => {
if (entry.isIntersecting) {
entry.target.classList.add('visible');
}
});
});
document.querySelectorAll('.lazy-load').forEach(element => observer.observe(element));
This API not only boosts performance by reducing the amount of JavaScript needed to control element visibility but also enhances user engagement by providing a smoother, lag-free browsing experience.
3. Notification API
The Notification API empowers web applications to engage users with desktop notifications, even when the browser is minimized or not actively in use. This functionality is crucial for real-time applications such as messaging apps, email clients, or any application that needs to alert users about important events or updates.
Notifications can be tailored with icons, interaction buttons, and even custom sounds, making them a versatile tool for increasing user engagement and retention.
if (Notification.permission === 'granted') {
new Notification('You have a new message!', {
icon: 'message-icon.png',
body: 'Hi there! You have received a new message.'
});
} else if (Notification.permission !== 'denied') {
Notification.requestPermission().then(permission => {
if (permission === 'granted') {
new Notification('Notifications are now enabled!');
}
});
}
Leveraging desktop notifications keeps users informed and engaged with your app in real time, significantly enhancing the application's usability and user satisfaction.
4. Speech Recognition API
The Speech Recognition API is a compelling tool for creating voice-driven interfaces in web applications. This API transforms spoken language into text, providing a basis for voice commands, speech-to-text services, and interactive voice response systems.
It opens up new avenues for accessibility, allowing users with disabilities or those who prefer voice interaction to engage with your web applications more effectively.
const recognition = new (window.SpeechRecognition || window.webkitSpeechRecognition)();
recognition.onresult = (event) => {
console.log('Recognized speech: ', event.results[0][0].transcript);
};
recognition.start();
By integrating voice capabilities, you can significantly enhance the accessibility and interactivity of your web applications, catering to a broader audience.
5. Web Share API
The Web Share API enables seamless sharing of links, text, or files directly to a user’s preferred social media or messaging apps from the web browser itself, bypassing the need for cumbersome copy-paste actions. This API is particularly beneficial for mobile users, offering a native-like sharing experience.
It supports a wide range of content types and is ideal for applications focused on content distribution, social interaction, or mobile commerce.
document.querySelector('#shareBtn').addEventListener('click', async () => {
try {
await navigator.share({
title: 'Check this out!',
text: 'Discover amazing content on our site.',
url: 'https://example.com'
});
console.log('Content shared successfully');
} catch (err) {
console.error('Failed to share content: ', err);
}
});
Integrating the Web Share API enhances user experience by providing a quick and easy way to share content, fostering greater content visibility and user interaction.
Conclusion
These underrated browser APIs showcase the breadth and depth of JavaScript’s capabilities in modern web development. From streamlining user interactions with the Clipboard API to enabling immersive experiences through the Intersection Observer and Notification APIs, these tools empower developers to craft innovative and efficient web applications. Explore these APIs in your next project, and watch as they transform your user experience and development process.
Follow Us:
Stay updated with our latest tips and tutorials by subscribing to our YouTube Channel.