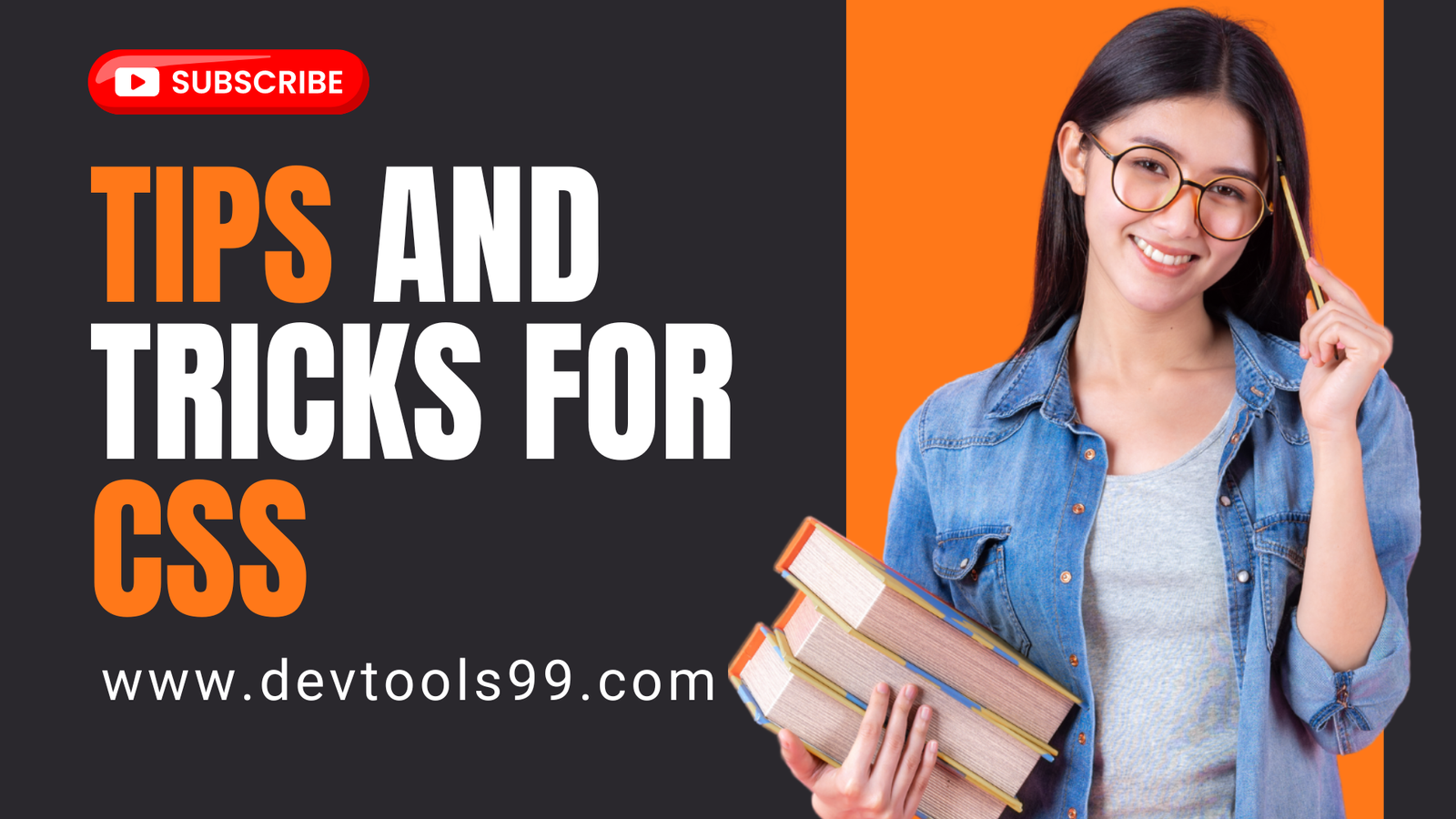
Tips and Tricks for Readable, Maintainable CSS
Writing CSS that is both readable and maintainable is crucial for long-term success in web development. Whether you're working alone or with a team, following best practices can save time, reduce errors, and make your stylesheets easier to understand and update. In this post, we'll explore various tips and tricks to help you achieve these goals.
1. Case Styles: Use kebab-case
When naming CSS classes, consistency is key. Avoid using PascalCase
or camelCase
for class names. Instead, prefer kebab-case
(also known as spinal-case) for better readability and consistency across your codebase.
.ButtonPrimary {} /* Avoid PascalCase */
.buttonPrimary {} /* Avoid camelCase */
.button-primary {} /* Prefer kebab-case */
2. Commenting: Keep It Clear and Useful
Commenting your CSS is important, but it's essential to use the right format. For single-line comments, use //
. For block comments, use /* ... */
. Clear and descriptive comments can make your code easier to understand, especially when revisiting it later or working in a team.
// This is a single-line comment
.nav {
color: #fff;
}
/*
* Header Section
*/
.header {
/* Main header styles */
color: #333;
}
3. Specifying Values: Simplify Where Possible
When resetting or assigning values to properties, there's no need to specify units for zero values. This not only simplifies your code but also makes it more efficient.
/* Not recommended */
*::after, *::before {
padding: 0px;
margin: 0px;
}
/* Recommended */
*::after, *::before {
padding: 0;
margin: 0;
}
4. Formatting: Pay Attention to Detail
Proper formatting in CSS is essential for readability. Always add a semicolon at the end of a property/value declaration. Additionally, use leading zeros for decimal values to maintain consistency.
/* Incorrect */
.button-primary {
margin: 0
}
/* Correct */
.button-primary {
margin: 0;
}
/* Incorrect */
.disabled {
opacity: .5;
}
/* Correct */
.disabled {
opacity: 0.5;
}
5. BEM: Block, Element, Modifier
The BEM (Block, Element, Modifier) methodology is a popular naming convention that improves the structure and clarity of your CSS, especially when using preprocessors like SCSS or SASS. It makes your code more modular and easier to maintain.
<div class="toast toast--error">
<p class="toast__message">
Message in here
</p>
</div>
Here's how you can structure the corresponding CSS:
.toast {
padding: 16px;
color: black;
&--error {
color: red;
}
&__message {
font-size: 16px;
}
}
6. DRY: Don’t Repeat Yourself
The DRY (Don't Repeat Yourself) principle is vital in programming, and CSS is no exception. Avoid redundant code by finding opportunities for reuse. For instance, you can combine similar styles into a single block and use modifiers to adjust specifics.
/* Without DRY principle */
.announcement-success {
padding: 32px;
border-radius: 16px;
background-color: lightgreen;
color: green;
}
.announcement-failed {
padding: 32px;
border-radius: 16px;
background-color: red;
color: white;
}
/* With DRY principle */
.announcement {
padding: 32px;
border-radius: 16px;
&--success {
background-color: lightgreen;
color: green;
}
&--failed {
background-color: red;
color: white;
}
}
Conclusion
Maintaining readable and maintainable CSS is not just about writing code that works; it's about writing code that others (and your future self) can easily understand and update. By following these tips, you can ensure that your stylesheets are clean, consistent, and scalable.
Adopting these best practices in your workflow will lead to a more efficient development process and a better overall product. Happy coding!
Follow Us:
Stay updated with our latest tips and tutorials by subscribing to our YouTube Channel.