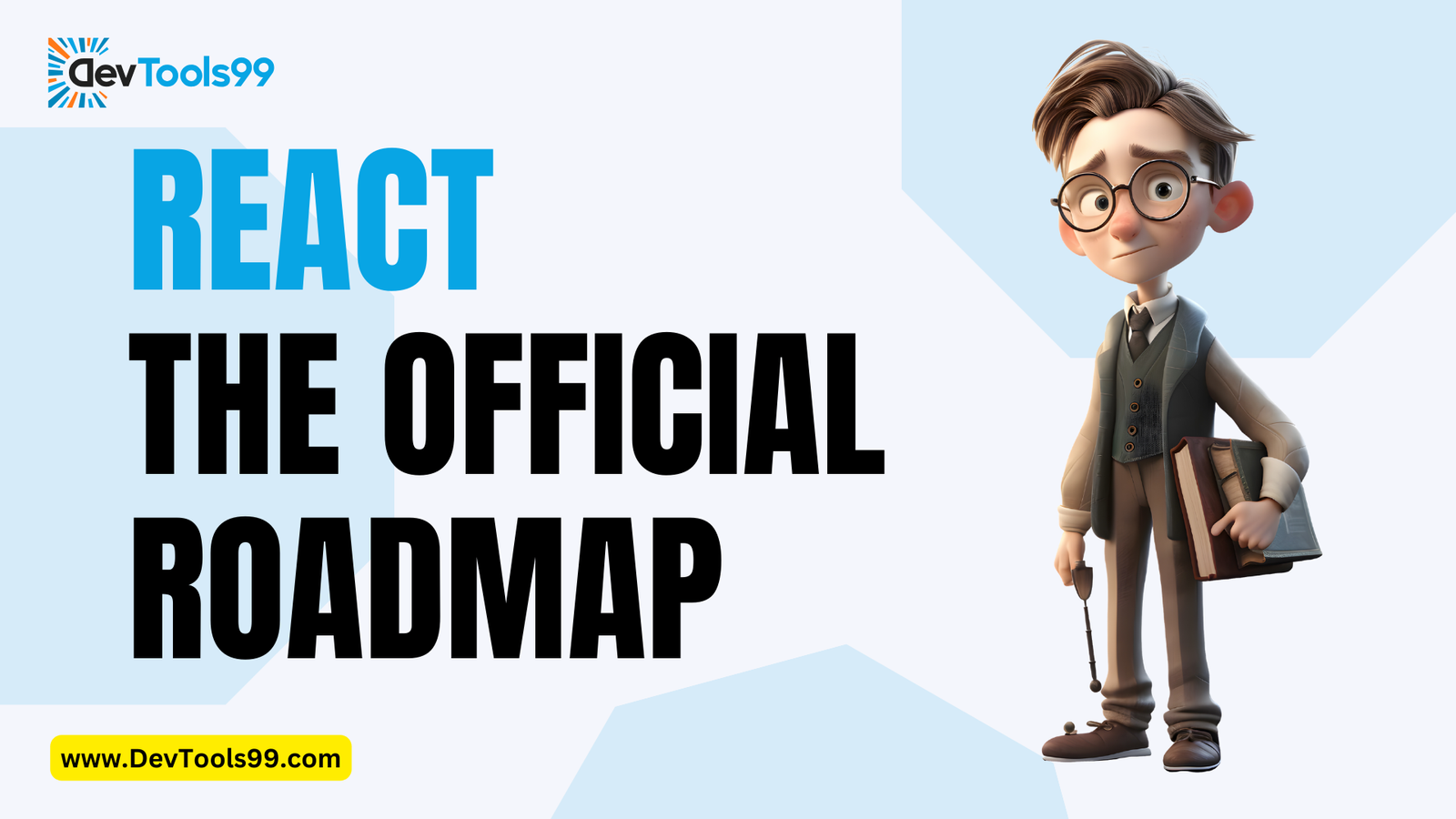
The Official Roadmap of React
The Prerequisites
Learning React is a great choice for Frontend Developers due to its large community and popularity in the industry. However, React is not a technology for absolute beginners. Here's what you will need to know before diving into React:
Basic HTML
- Common Tags
- Attributes
- File Structure
Explanation: HTML is the foundation of web development. You should know how to use common tags like <div>
, <p>
, and <a>
, understand how to use attributes to add additional information to tags, and understand the basic structure of an HTML file.
Basic CSS
- Applying Styles
- Flexbox Layouts
- Media Queries
Explanation: CSS is used to style your HTML content. You need to know how to apply styles to HTML elements, use Flexbox for layout design, and apply media queries for responsive design.
Good Knowledge of JavaScript (ES6)
- Variables (
let
,const
) - Loops & Conditionals
- Arrow Functions
- Arrays, Objects
- Imports and Exports
Explanation: JavaScript is essential for React. Understanding ES6 syntax is crucial. You should be comfortable with declaring variables using let
and const
, using loops and conditionals to control flow, writing concise functions with arrow syntax, manipulating arrays and objects, and modularizing your code with import/export statements.
How to use NPM
- Installing packages
- Managing dependencies
Explanation: NPM (Node Package Manager) is used to manage libraries and packages in your projects. To install a package, you can use the command npm install package-name
. Dependencies are listed in the package.json
file and can be managed by updating, removing, or adding new packages using NPM commands.
npm install react
Run the above command to install React.
The First Steps
Understanding the base concepts of React apps is crucial. Start by creating a simple application, studying the folder structure, and grasping the concept of components. Your initial path should include:
create-react-app
Explanation: create-react-app
is a command-line tool that sets up a new React project with a sensible default configuration. To create a new project, run:
npx create-react-app my-app
This command will create a new directory called my-app
with all the necessary files and dependencies.
Hooks: useState & useEffect
Explanation: Hooks are functions that let you use state and other React features in functional components. useState
allows you to add state to a functional component, and useEffect
lets you perform side effects in your components (such as fetching data or directly interacting with the DOM).
Components
Explanation: Components are the building blocks of a React application. They can be functional or class-based.
Functional Components
Explanation: Functional components are simple JavaScript functions that return React elements. They are typically easier to write and understand than class components.
Props & State
Explanation: Props are used to pass data from one component to another. State is used to manage data that changes over time within a component.
JSX
Explanation: JSX is a syntax extension for JavaScript that allows you to write HTML-like code within JavaScript. It makes writing React components more intuitive.
Conditional Rendering
Explanation: Conditional rendering in React allows you to render different UI elements based on certain conditions. This can be done using JavaScript conditional statements within your JSX.
Lists & Keys
Explanation: Rendering lists of data is common in React. Keys help React identify which items have changed, are added, or are removed, which optimizes rendering performance.
Events & Input Handling
Explanation: Managing user interactions and form inputs involves handling events such as clicks, form submissions, and input changes. React provides a way to handle these events within your components.
The React Ecosystem
Since React is a library and not a full-fledged framework, you will need to work with various other libraries to build complete applications. Here's the path to take:
Routing
Explanation: Routing in React allows you to navigate between different pages or views in your application. React Router
is a popular library for handling client-side routing.
React Router
Explanation: React Router
provides a way to manage routes and navigation in your React application. To use React Router, install it using NPM:
npm install react-router-dom
State Management
Explanation: Managing state in larger applications can become complex. React provides tools like the Context API and libraries like Redux to help manage state efficiently.
Context API
Explanation: The Context API allows you to share state across multiple components without prop drilling.
Redux
Explanation: Redux is a state management library for managing and centralizing application state. To use Redux, install it using NPM:
npm install redux react-redux
Styling
Explanation: There are various ways to style React components, including CSS-in-JS libraries and utility-first CSS frameworks.
Styled Components
Explanation: Styled Components is a library that allows you to write CSS in JavaScript. Install it using NPM:
npm install styled-components
Tailwind CSS
Explanation: Tailwind CSS is a utility-first CSS framework for rapidly building custom designs. To use Tailwind CSS in a React project, follow the installation guide on the official website.
HTTP
Explanation: Making HTTP requests in React is essential for interacting with APIs. You can use the native Fetch API or libraries like Axios.
Using Fetch In React
Explanation: The Fetch API is a built-in JavaScript method for making HTTP requests. Example:
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => console.log(data));
Axios
Explanation: Axios is a popular HTTP client for making requests. Install it using NPM:
npm install axios
Forms
Explanation: Managing form state and validation is crucial in any web application.
Hook Form Handling
Explanation: Libraries like React Hook Form simplify form handling using hooks. Install it using NPM:
npm install react-hook-form
Formik
Explanation: Formik is a library for building and managing forms in React. Install it using NPM:
npm install formik
The Advanced Topics
Once you've mastered the basics, move on to learning these advanced topics:
Advanced Hooks
useRef
: Accessing and manipulating DOM elements.useMemo
: Memoizing expensive calculations.useCallback
: Memoizing functions to optimize performance.
Refs
Explanation: Refs provide a way to access DOM nodes or React elements created in the render method.
Error Boundaries
Explanation: Error boundaries are React components that catch JavaScript errors anywhere in their child component tree, log those errors, and display a fallback UI.
Higher Order Components (HOCs)
Explanation: HOCs are functions that take a component and return a new component with added functionality.
Practice!
Explanation: Continuously practice by building projects and adding them to your portfolio. Showcase your skills to impress potential employers or clients.
Follow Us:
Stay updated with our latest tips and tutorials by subscribing to our YouTube Channel.