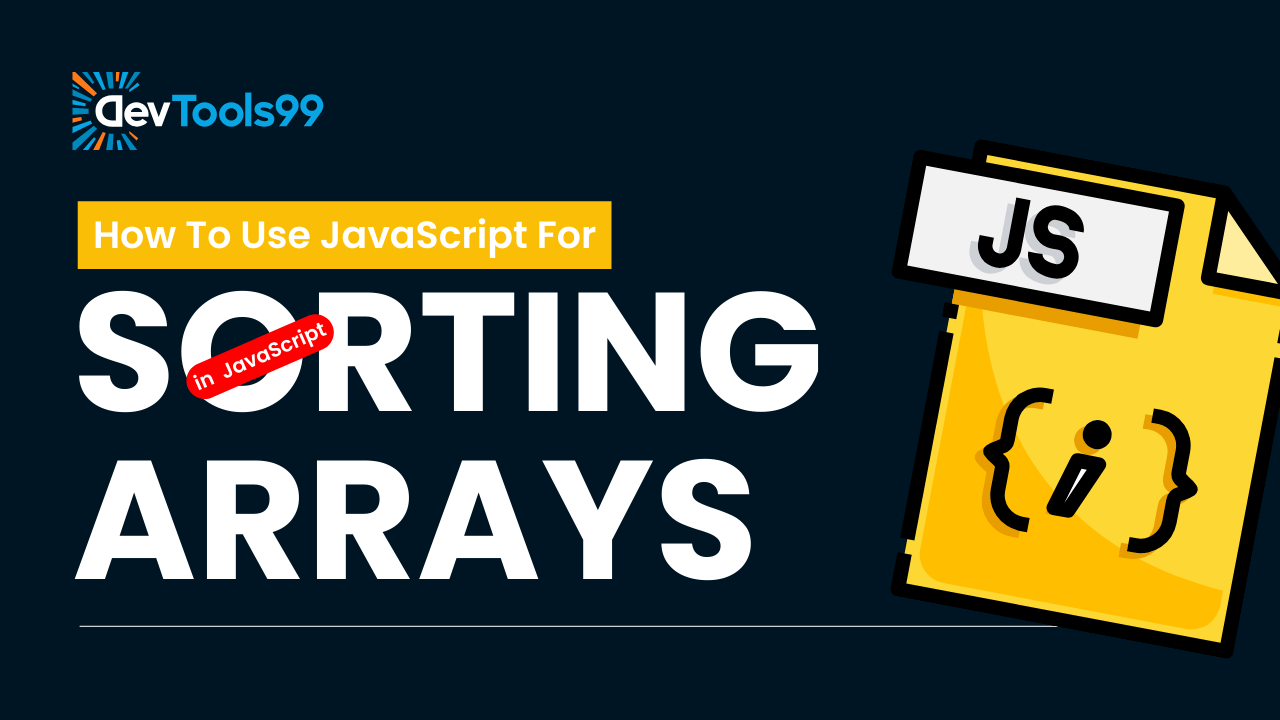
Sorting Arrays in JavaScript
Sorting arrays is a common task in JavaScript, and it can sometimes be tricky depending on the type of data you're dealing with. In this tutorial, we’ll cover various scenarios for sorting arrays, including arrays containing strings, numbers, strings with numbers, strings with long numbers, and objects. By the end of this guide, you'll have a solid understanding of how to sort different types of arrays and tackle sorting challenges effectively.
1. Sorting Strings
JavaScript's sort()
method sorts strings alphabetically by default, based on their Unicode values. For example, the array ["banana", "apple", "cherry"]
will be sorted as ["apple", "banana", "cherry"]
. However, case-sensitivity affects sorting; uppercase letters will precede lowercase ones.
const fruits = ["banana", "Apple", "cherry"];
fruits.sort();
console.log(fruits); // ["Apple", "banana", "cherry"]
If you want case-insensitive sorting, you can use a custom comparison function:
fruits.sort((a, b) => a.localeCompare(b, undefined, { sensitivity: 'base' }));
console.log(fruits); // ["Apple", "banana", "cherry"]
2. Sorting Numbers
By default, sort()
treats numbers as strings, which can lead to unexpected results:
const numbers = [10, 2, 30];
numbers.sort();
console.log(numbers); // [10, 2, 30]
To sort numbers properly, you must provide a comparison function:
numbers.sort((a, b) => a - b); // Ascending order
console.log(numbers); // [2, 10, 30]
numbers.sort((a, b) => b - a); // Descending order
console.log(numbers); // [30, 10, 2]
3. Sorting Strings with Numbers
When strings contain numbers (e.g., "item1"
, "item10"
), sorting them alphabetically may not produce the desired order. Instead, extract the numeric portion and sort numerically:
const items = ["item1", "item10", "item2"];
items.sort((a, b) => {
const numA = parseInt(a.match(/\d+/)[0]);
const numB = parseInt(b.match(/\d+/)[0]);
return numA - numB;
});
console.log(items); // ["item1", "item2", "item10"]
4. Sorting Strings with Long Numbers
For strings with long numeric values (e.g., "item100"
, "item20"
), you can use the same approach with regular expressions:
const items = ["item100", "item20", "item3"];
items.sort((a, b) => {
const numA = parseInt(a.match(/\d+/)[0]);
const numB = parseInt(b.match(/\d+/)[0]);
return numA - numB;
});
console.log(items); // ["item3", "item20", "item100"]
5. Sorting Objects
To sort an array of objects by a specific property, such as id
, use a comparison function in the sort()
method:
const users = [
{ id: 3, name: "Alice" },
{ id: 1, name: "Bob" },
{ id: 2, name: "Charlie" }
];
users.sort((a, b) => a.id - b.id);
console.log(users);
// [
// { id: 1, name: "Bob" },
// { id: 2, name: "Charlie" },
// { id: 3, name: "Alice" }
// ]
Conclusion
Sorting arrays in JavaScript can be straightforward or complex depending on the type of data you’re working with. By understanding how sort()
works and using custom comparison functions, you can effectively sort strings, numbers, and objects in any scenario. Experiment with these methods to master array sorting and handle any data structure with ease!
Follow Us:
Stay updated with our latest tips and tutorials by subscribing to our YouTube Channel.