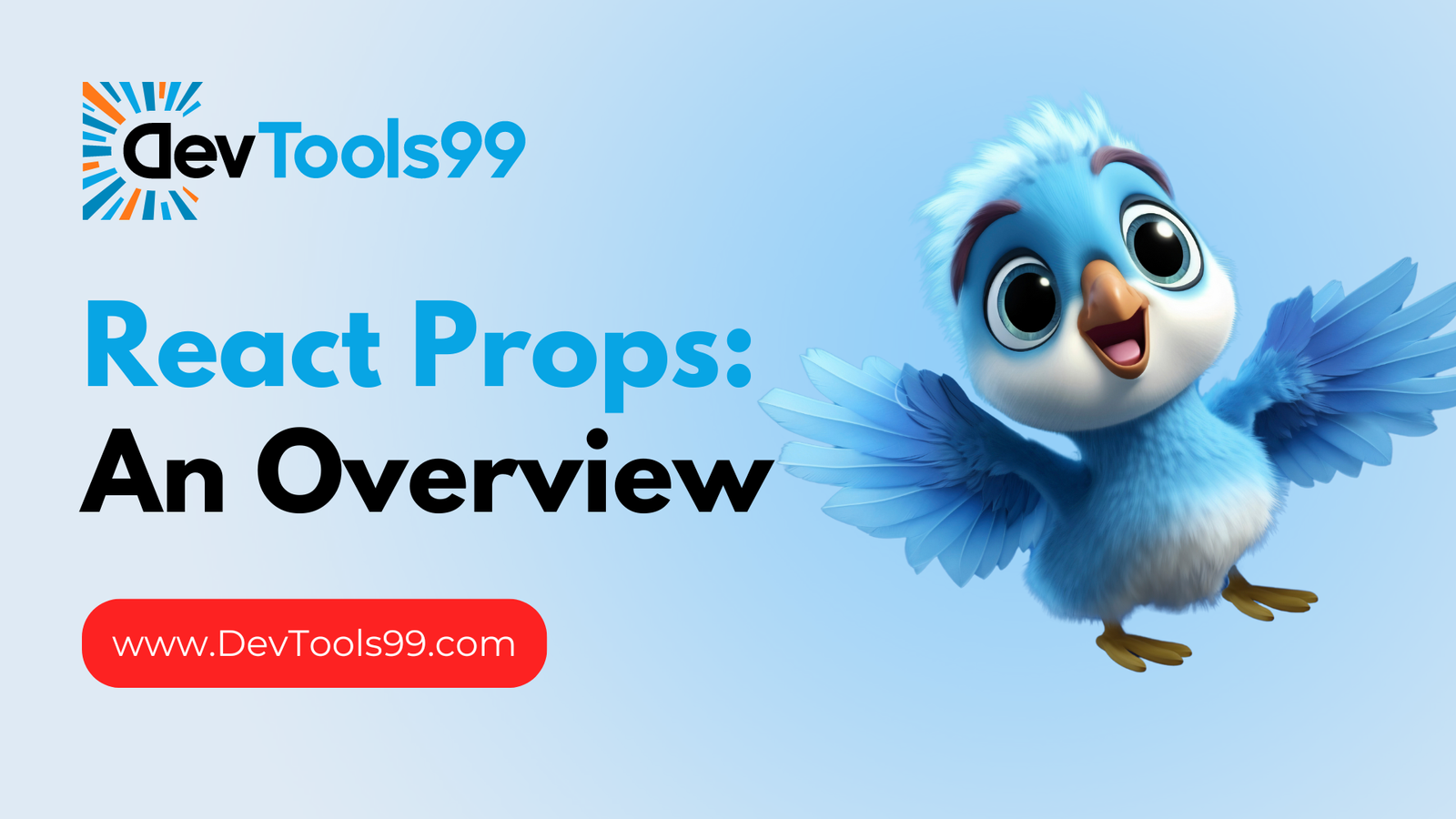
Understanding React JS Props
React is a popular JavaScript library for building user interfaces, particularly single-page applications. One of the core concepts in React is the use of props. In this blog post, we will explore what props are, their purpose, and how to use them effectively in your React applications.
What are React Props?
Props, short for properties, are arguments passed into React components. They allow you to pass data from one component to another, making your components more dynamic and reusable. Props are passed to components via HTML attributes and are similar to function arguments in JavaScript or attributes in HTML.
One important aspect of React's data flow is that it is unidirectional, meaning data flows from parent to child components only. This design makes it easier to understand and predict how data moves through your application.
For example, consider a parent component that holds the state and passes it down to child components via props:
function ParentComponent() {
const [data, setData] = useState('Hello, world!');
return <ChildComponent message={data} />;
}
function ChildComponent(props) {
return <p>{props.message}</p>;
}
Purpose of Props
Props serve several key purposes in React:
- Data Passing: They are used to pass data between React components. This allows you to build complex interfaces by composing simple, reusable components.
- Function Arguments: Props are passed to the component in the same way as arguments passed in a function. This makes the component flexible and reusable with different sets of data.
- Immutable Data: Props are immutable, meaning you cannot modify the props from inside the component. This ensures data consistency and predictability in your application, as components rely on receiving consistent data from their parents.
For example, you can pass user data from a parent component to a child component to display user information:
function UserProfile(props) {
return (
<div>
<h2>User: {props.name}</h2>
<p>Age: {props.age}</p>
</div>
);
}
function App() {
return <UserProfile name="Alice" age={25} />;
}
Props Example
To send props into a component, use the same syntax as HTML attributes. For example, let's add a "brand" attribute to a Laptop
element:
const myElement = <Laptop brand="Acer" />;
The component receives the argument as a props object:
function Laptop(props) {
return <h2>This is a {props.brand} Laptop</h2>;
}
This way, the Laptop
component can render different content based on the props it receives, making it reusable for different brands.
Passing Data
Props are also how you pass data from one component to another, similar to passing parameters in functions. For example, you can create a variable named webName
and send it to the WebApp
component:
const webName = "Live in my Server";
function WebApp(props) {
return <h2>{props.webName}</h2>;
}
const myApp = <WebApp webName={webName} />;
This approach allows you to keep your components decoupled and focused on their specific tasks, improving code maintainability and readability.
Default Props
It's not necessary to always pass props from parent components. You can also set default props directly on the component. This is useful for ensuring your components have default values when no props are provided:
import React from 'react';
function WebApp(props) {
return <h2>Welcome To {props.name}</h2>;
}
WebApp.defaultProps = {
name: 'Code.clash'
};
export default WebApp;
Default props are helpful in scenarios where you want to provide fallback values to ensure your component behaves correctly even if some props are not passed:
function Greeting(props) {
return <h1>Hello, {props.name}!</h1>;
}
Greeting.defaultProps = {
name: 'Guest'
};
// Usage
<Greeting />; // Renders: Hello, Guest!
<Greeting name="Alice" />; // Renders: Hello, Alice!
Props Are Read-Only
One important thing to remember is that React props are read-only. You will get an error if you try to change their value. This immutability ensures that data flows in a predictable manner and helps maintain the integrity of the component's state.
Attempting to modify props directly will result in an error. Instead, you should use state for data that needs to change over time:
function Counter(props) {
const [count, setCount] = useState(props.initialCount);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
Counter.defaultProps = {
initialCount: 0
};
// Usage
<Counter initialCount={10} />; // Starts with count 10
Best of Luck! Understanding and using props effectively can greatly enhance your React development skills. Keep practicing and exploring more advanced concepts as you progress.
Follow Us:
Stay updated with our latest tips and tutorials by subscribing to our YouTube Channel.