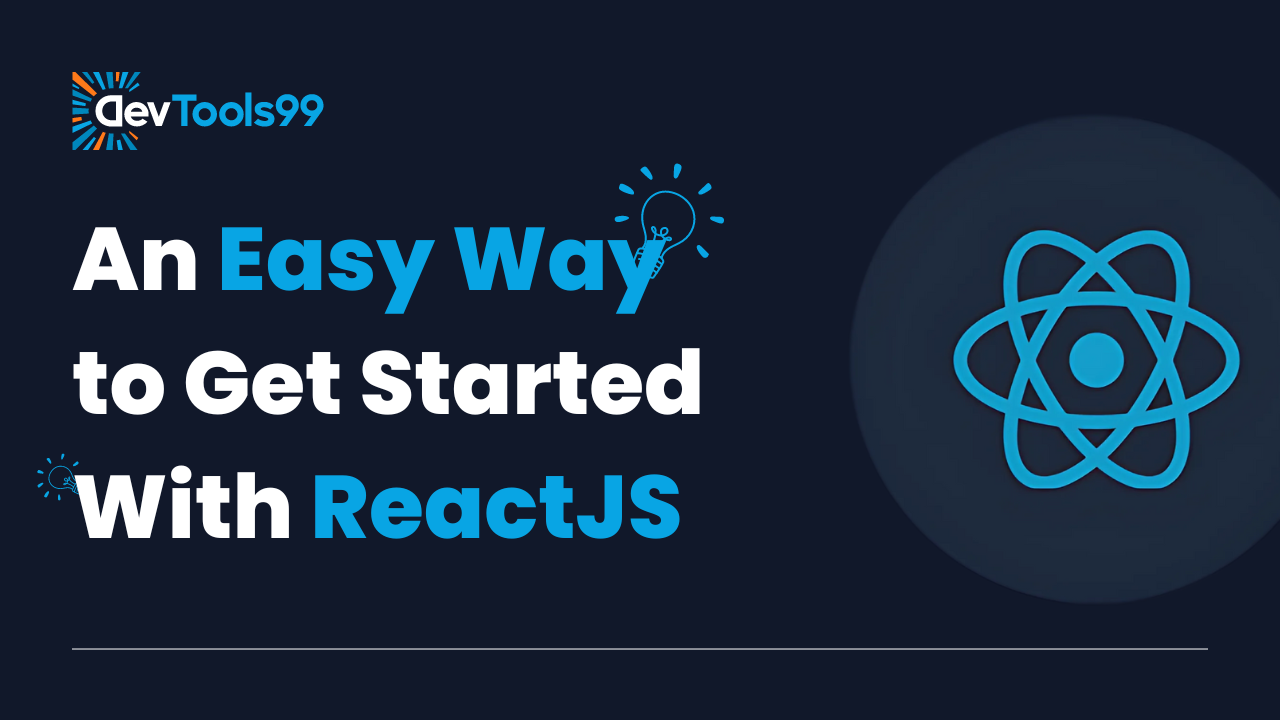
An Easy Way to Get Started With ReactJS
ReactJS is one of the most popular JavaScript libraries for building user interfaces. Whether you’re new to front-end development or looking to switch from another framework, React's modular and flexible structure makes it easier to build scalable applications. In this guide, we'll walk through some key concepts to help you get started with ReactJS.
1. JSX
JSX (JavaScript XML) is a syntax extension for JavaScript that looks similar to HTML but comes with enhanced capabilities. It allows you to describe the UI components and mix them with JavaScript logic. Although it resembles HTML, JSX is stricter and must follow proper formatting rules like self-closing tags, camelCase attributes, and valid JavaScript expressions.
const element = <h1>Hello, world!</h1>;
Using JSX makes your code more declarative and easy to understand. While it is optional, most React developers find it the easiest way to build UIs.
2. Components
In React, the entire UI is broken down into components. Components are reusable building blocks of React apps. You can think of them as custom HTML elements that render specific parts of your UI.
function HeroSection() {
return <div>Welcome to the Hero Section</div>;
}
You can also nest components inside one another to create a more structured UI. For example, the HeroSection
component can be reused multiple times throughout your app to maintain consistency in design.
3. Styling
There are several ways to style React components, but the most common are using CSS classes or inline styles:
- CSS Classes: Use the
className
attribute instead of the standardclass
attribute used in HTML to apply external CSS styles. - Inline Styles: You can apply styles inline using a JavaScript object with camelCased CSS properties.
const styles = { color: 'blue', fontSize: '20px' };
<h1 style={styles}>Styled Text</h1>
Both approaches can be used depending on the complexity and reusability of your styles.
4. Conditional Rendering
In React, you can control what is rendered based on certain conditions. This is often done using JavaScript logic such as the &&
operator or ternary operators.
const isLoggedIn = true;
return (
<div>
{isLoggedIn ? <p>Welcome back!</p> : <p>Please sign in.</p>}
</div>
);
This is particularly useful when you need to show different UIs based on certain user actions or states.
5. Rendering a List
Rendering lists of items is a common task in React. You can iterate through an array and render a component for each item. However, React requires you to include a unique key
for each list item to help identify which items have changed, been added, or removed.
const items = ['Apple', 'Banana', 'Cherry'];
return (
<ul>
{items.map((item, index) => (
<li key={index}>{item}</li>
))}
</ul>
);
Without unique keys, React would have difficulty keeping track of changes in the list, leading to unexpected behavior.
6. Events Handling
React provides built-in event handling, making it easy to capture user interactions like clicks, form submissions, or input changes. The events in React are named using camelCase (e.g., onClick
, onSubmit
), and they handle these events similarly to vanilla JavaScript.
function handleClick() {
alert('Button clicked');
}
return <button onClick={handleClick}>Click Me</button>;
Event handling in React allows you to capture and respond to user input dynamically.
7. Hooks
Hooks are functions introduced in React 16.8 that allow you to use state and other React features in functional components. They have replaced the need to write class components in many cases, making React code easier to manage and scale.
useState
- manages component state.useEffect
- performs side effects like fetching data or updating the DOM.useContext
- allows for global state management across components.
const [count, setCount] = useState(0);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
By using hooks, you can maintain cleaner and more readable code while achieving advanced functionality in your app.
8. Sharing Data Between Components
Data in React is passed down from parent to child components via props
. When two components need to share data, you can "lift" the state up to their common parent and pass it down to the relevant components as props. This allows components to stay in sync while keeping the code modular.
function ParentComponent() {
const [count, setCount] = useState(0);
return (
<div>
<ChildComponent count={count} increment={() => setCount(count + 1)} />
</div>
);
}
This ensures that data is shared between components without the need for a centralized state management system in smaller apps.
Conclusion
ReactJS is a powerful library that gives developers a structured way to build efficient and scalable UIs. By understanding JSX, components, state, hooks, and other key concepts, you can quickly get up and running with React. The more you practice, the easier it will become to build complex applications using React’s modular approach. Once comfortable with the basics, explore more advanced features like Context, Portals, and React Router to further enhance your skills.
Follow Us:
Stay updated with our latest tips and tutorials by subscribing to our YouTube Channel.