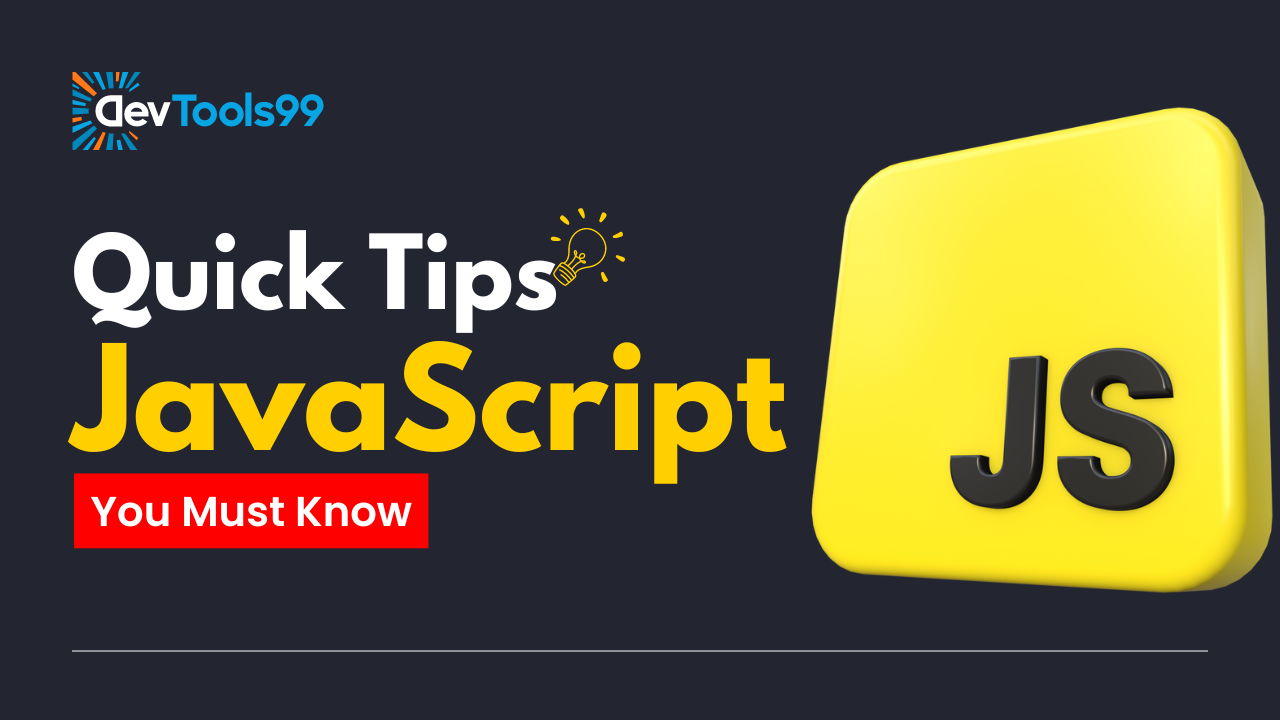
Quick JavaScript Tips for Developers
JavaScript is a versatile language that allows developers to manipulate data with ease. Below are some quick tips that will help you improve your coding efficiency, particularly when working with arrays, data types, and numbers. These simple snippets can save you time and simplify your code.
1. Filter Unique Values
One of the most common tasks when working with arrays is ensuring that all values are unique. Using the Set
object is a quick and easy way to remove duplicates.
const uniqueArray = [...new Set(array)];
The Set
object stores only unique values, and by spreading it into an array, you get back a new array with duplicates removed.
2. Convert to Boolean
Sometimes you need to check the truthiness of a value. In JavaScript, this is done using Boolean()
or a double negation (!!
). Both methods will convert a value to its boolean equivalent.
const isTrue = Boolean(value);
// or
const isTrue = !!value;
Falsy values such as 0
, null
, undefined
, and ""
(empty string) will be converted to false
, while all other values will return true
.
3. Convert to String
Converting a value to a string is often necessary when dealing with user interfaces or displaying data. The String()
function or toString()
method converts any type to a string.
const strValue = String(value);
// or
const strValue = value.toString();
This method ensures that numbers, booleans, or even objects are transformed into string format, making them suitable for display or further string manipulation.
4. Convert to Integer
To convert strings or floating-point numbers to integers, you can use parseInt()
. Another alternative is using the unary plus operator (+value
), though this might leave you with a float if the value isn't already an integer.
const intValue = parseInt(value);
// or
const intValue = +value;
Keep in mind that parseInt()
allows you to specify the base of the number, while +value
is a more implicit conversion and can result in decimals if the input is a float.
5. Convert Float to Integer
When converting floats to integers, you may need to decide how to handle the decimal part. You can round down, round up, or round to the nearest integer using the Math
object’s built-in methods.
const floored = Math.floor(value); // round down
const ceiled = Math.ceil(value); // round up
const rounded = Math.round(value); // round to nearest integer
Each of these methods has its use case depending on whether you need precision or just an approximation.
6. Remove Last Digits from a Number
To truncate the last digit(s) of a number, a handy trick is dividing by 10 and using Math.trunc()
to remove any fractional parts.
const truncated = Math.trunc(value / 10);
This is particularly useful when you need to reduce the precision of a number or cut off unwanted digits from a longer number.
7. Get the Last Item in an Array
Accessing the last element in an array can be done by using the array's length minus one. This gives you direct access to the last index.
const lastItem = array[array.length - 1];
This method is concise and works reliably even if the array length changes dynamically during execution.
8. Truncate an Array
If you need to shorten an array to a specific length, you can do so by directly modifying its length
property.
array.length = desiredLength;
This operation will cut off elements that exceed the specified length, allowing for quick array truncation.
Conclusion
These quick JavaScript tips are useful for various tasks such as manipulating arrays, converting between data types, and rounding numbers. Whether you're filtering unique values or working with number conversions, these tips can help you write cleaner, more efficient code. Bookmark these for future reference to streamline your development workflow!
Follow Us:
Stay updated with our latest tips and tutorials by subscribing to our YouTube Channel.