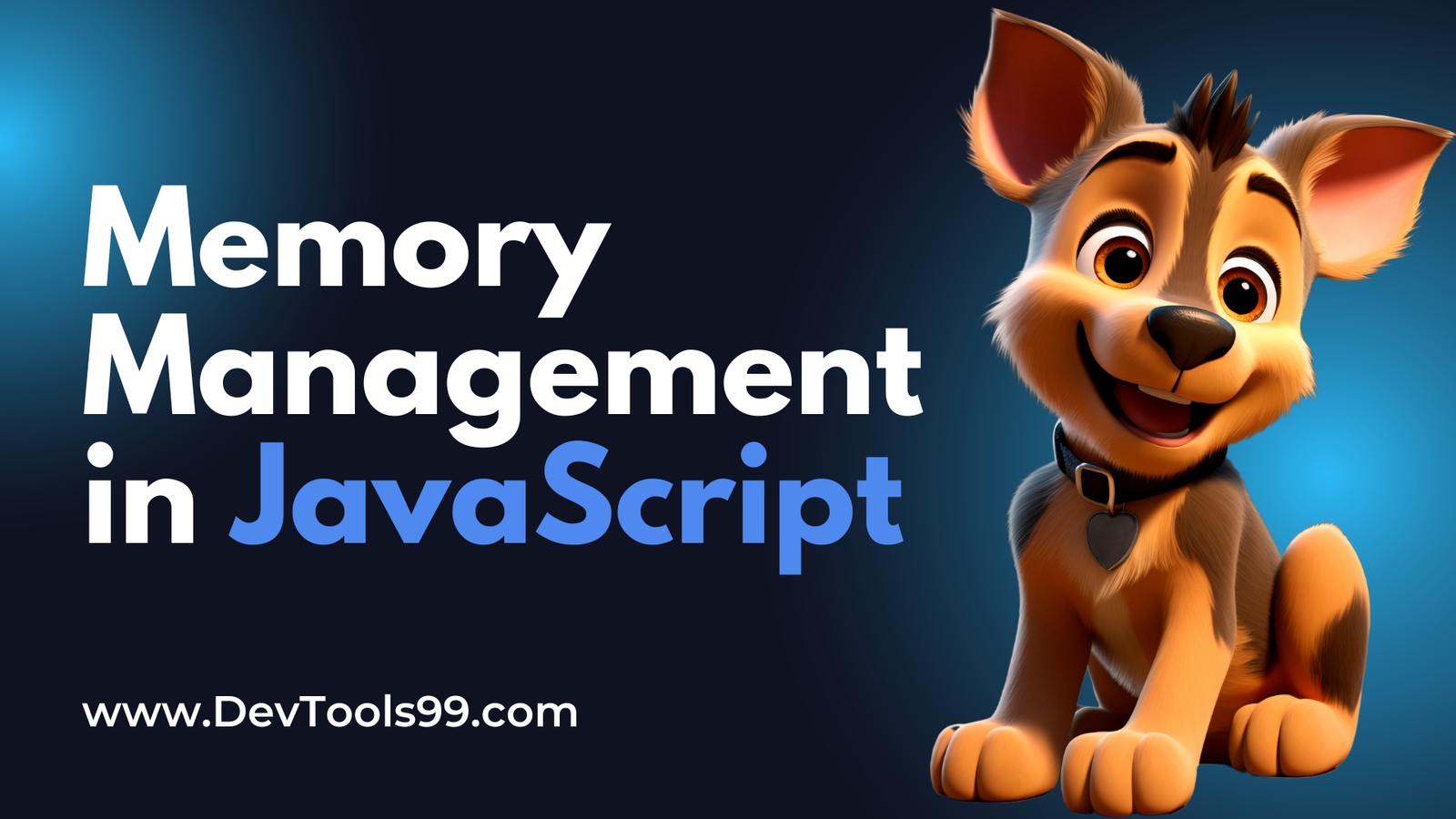
Memory Management in JavaScript.
Memory management in JavaScript is the process of allocating, using, and releasing memory resources efficiently to ensure the smooth functioning of JavaScript applications. Both browsers and Node.js handle memory management, but they do so in slightly different environments. Let's explore memory management in both contexts and provide code examples to illustrate the concepts.
Memory Management in Browsers:
In the browser, JavaScript runs in a sandboxed environment and is responsible for managing memory used by the web page.
Garbage Collection: JavaScript uses automatic garbage collection to reclaim memory occupied by objects that are no longer reachable or in use. Garbage collection is performed by the JavaScript engine, and developers don't need to manually free memory.
Memory Management in Node.js:
Node.js, being a server-side runtime environment, also has its memory management, but it operates differently from browsers.
V8 Heap: Node.js uses the V8 JavaScript engine, which has its memory heap. Memory allocation and garbage collection are handled by V8. Node.js provides some additional features and APIs for managing memory efficiently.
Managing Streams:
Node.js often deals with I/O operations and streams, which require special care in managing memory. Developers should use streams effectively to avoid memory leaks.
Buffer and Buffer Pools:
Node.js provides a Buffer class for handling binary data efficiently. Buffers are allocated outside the JavaScript heap and can be used for reading, writing, and manipulating binary data. Node.js also employs a buffer pool to manage memory allocation for buffers, reducing memory fragmentation.
Memory Leaks:
In both browser and Node.js environments, memory leaks can occur when references to objects or resources are unintentionally retained, preventing them from being garbage collected. Developers need to be vigilant and use tools like memory profilers to identify and address memory leaks.
In summary, memory management in JavaScript involves automatic garbage collection, proper handling of I/O operations and streams, effective use of buffers, and vigilance against memory leaks. Both browsers and Node.js provide mechanisms to manage memory efficiently, but developers must understand these concepts and use them effectively to build robust applications.
Like, Share and Subscribe #DevTools99 for more useful videos, tools info and #tutorials . Thank you!