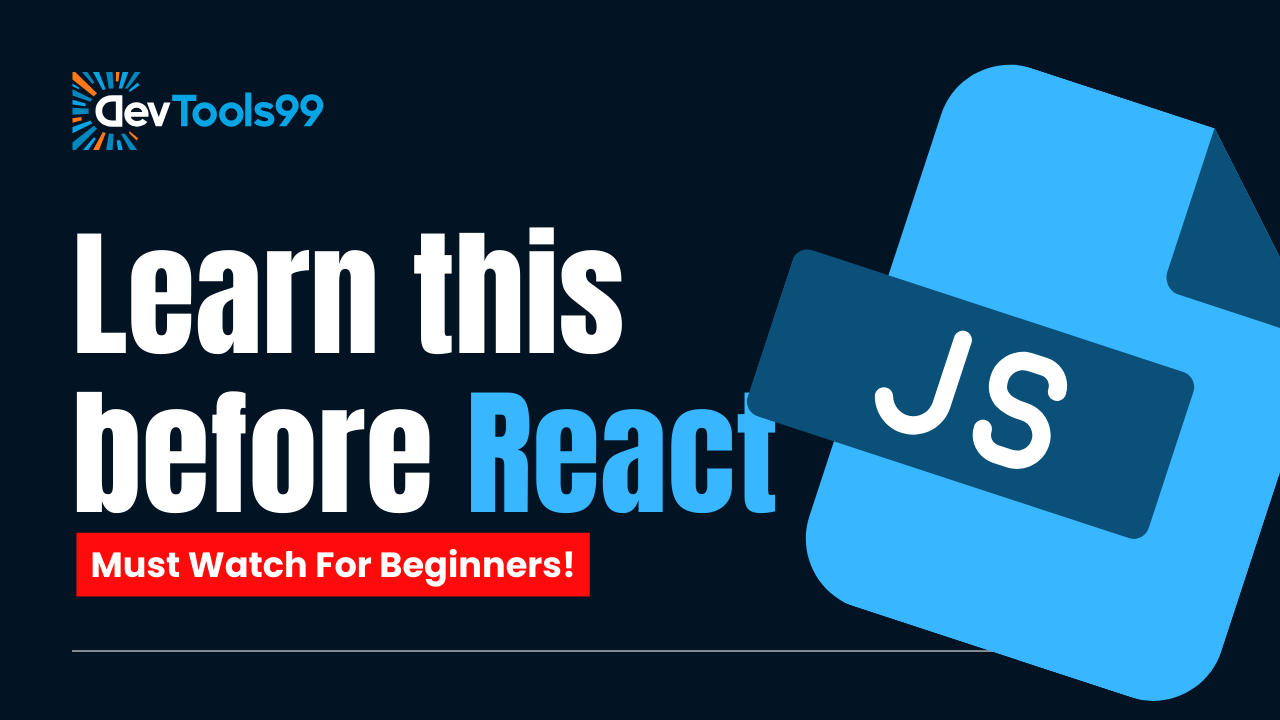
Learn This Before React
React.js and React Native are among the most popular tools for building modern web and mobile applications. However, to truly excel in these frameworks, a strong grasp of fundamental JavaScript concepts is essential. These concepts act as building blocks, enabling you to write cleaner, more efficient code while navigating React's syntax and features with ease.
Why Master JavaScript Before React?
React heavily relies on JavaScript for manipulating the DOM, handling events, and managing data flow. Without a solid foundation in JavaScript, you may find React's learning curve steeper than necessary. Mastering these essential concepts will not only make React easier to understand but also empower you to solve common challenges quickly and efficiently.
.map()
& .filter()
- Transforming and Filtering Data
The .map()
and .filter()
methods are indispensable when working with arrays in JavaScript:
.map()
: This method applies a function to every element in an array, transforming the data and returning a new array. For instance, if you need to double every number in an array or create JSX elements from a list,.map()
is your go-to tool..filter()
: Use this method to create a new array with elements that meet specific criteria. For example, filtering out users older than a certain age or isolating products in stock is made simple with.filter()
.
slice()
& splice()
- Handling Array Segments
Both slice()
and splice()
let you manipulate arrays, but their use cases differ:
slice()
: This non-destructive method allows you to extract a portion of an array without altering the original. It's perfect when you want to create a subset, such as displaying only the first 5 results of a list.splice()
: Unlikeslice()
, this method directly modifies the original array. Use it to add, remove, or replace elements, such as updating a shopping cart in a React app.
concat()
- Combining Arrays
The .concat()
method merges multiple arrays into one, leaving the originals untouched. Whether you’re combining product lists or merging user data, this method ensures data integrity while providing a seamless way to manage collections.
find()
& findIndex()
- Searching Arrays
Efficiently searching for data in arrays is crucial in React apps, and these methods make it easy:
find()
: Returns the first element that satisfies a given condition. For instance, finding a user by ID in a user list.findIndex()
: Similar tofind()
, but instead of the element, it returns its index, helping you locate and update specific items.
Destructuring - Simplifying Data Access
Destructuring allows you to unpack values from arrays or objects into variables with minimal code. In React, this is especially handy for extracting props or state values. For example:
const { name, age } = user; // Extracts 'name' and 'age' from a 'user' object
This approach makes your code cleaner and more readable, reducing redundancy and potential errors.
Rest & Spread Operators - Versatile Tools
The rest and spread operators are incredibly powerful for managing and manipulating data in JavaScript:
- Rest Operator: Collects multiple values into a single array or object. It's especially useful for handling dynamic arguments in functions or grouping elements.
- Spread Operator: Expands elements of an array or object into individual components. Commonly used for cloning arrays or objects and merging data structures effortlessly.
In React, these operators play a vital role in tasks like copying state, merging props, or handling dynamic data more efficiently.
Promises - Managing Asynchronous Code
Promises are a cornerstone of modern JavaScript, essential for handling asynchronous operations such as API requests or file uploads. They represent a value that may be available immediately, in the future, or never, and operate in one of three states: pending, fulfilled, or rejected.
Using methods like .then()
, .catch()
, and .finally()
, promises provide a structured way to manage success and error states. In React, understanding promises is critical for handling data fetching, managing side effects, and ensuring a seamless user experience.
Conclusion
By mastering these JavaScript concepts, you’ll build a strong foundation for working with React. From manipulating arrays to managing asynchronous code, these skills simplify the process of creating dynamic, responsive applications. Whether you're building a simple app or scaling to a complex project, these tools will save time and make your code cleaner and more maintainable.
Begin your journey by focusing on these fundamentals, and React will become far more approachable. With practice, these techniques will help you confidently tackle real-world challenges. Happy coding!
Follow Us:
Stay updated with our latest tips and tutorials by subscribing to our YouTube Channel.