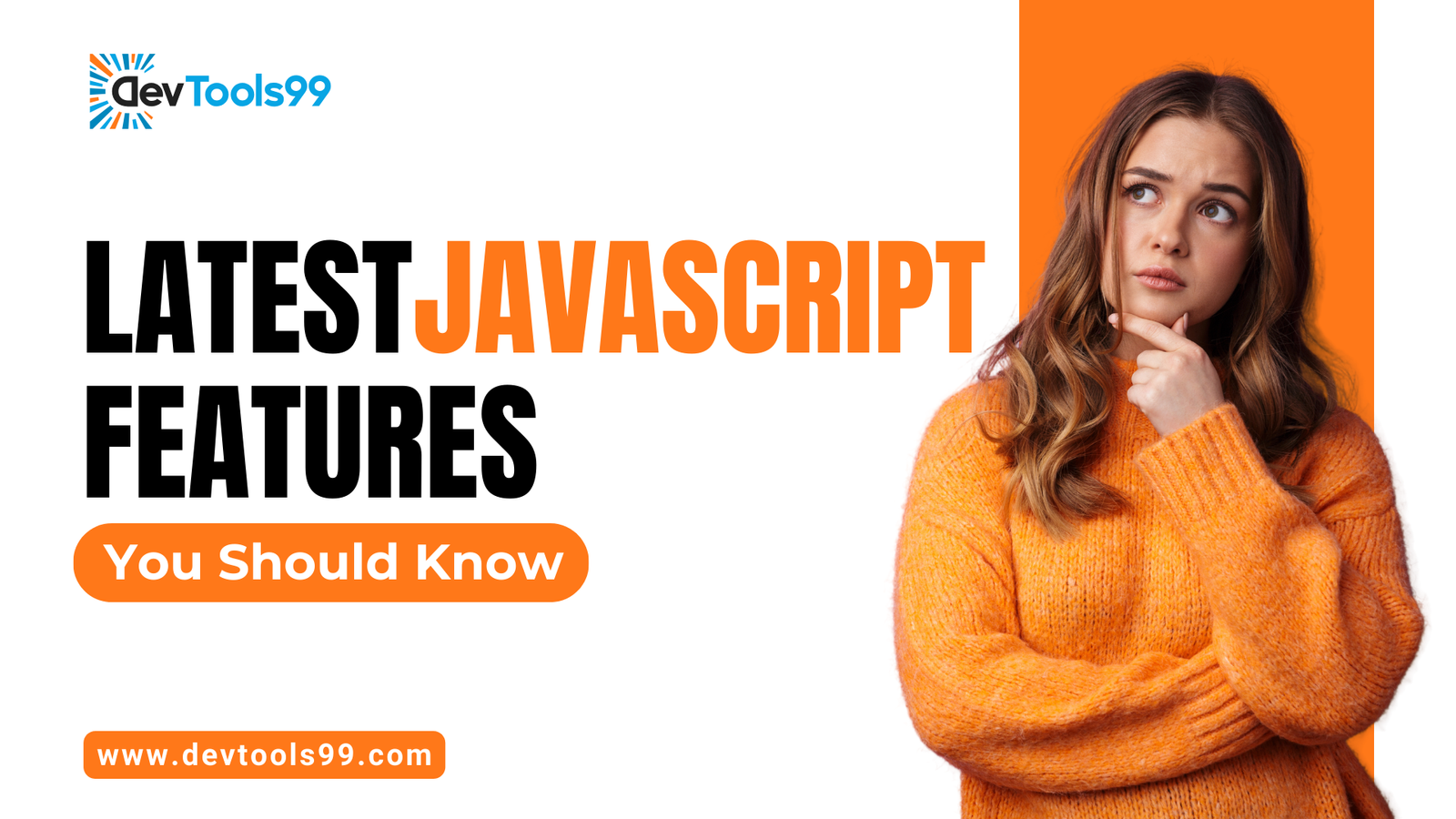
Latest JS Feature
JavaScript continues to evolve, bringing new methods and features that enhance the way we write and manipulate code. In this blog post, we'll explore some of the latest JavaScript features that you should be aware of. These new methods provide powerful tools for working with arrays in more efficient and intuitive ways.
findLast
The findLast()
method looks at an array from the end and gives you the value of the first element that meets a certain condition.
Example:
const numbers = [1, 2, 3, 4, 5, 6];
const lastEven = numbers.findLast(num => num % 2 === 0);
console.log(lastEven); // Output: 6
findLastIndex
The findLastIndex()
method finds the position in the array of the last element that meets a certain condition.
Example:
const numbers = [1, 2, 3, 4, 5, 6];
const lastEvenIndex = numbers.findLastIndex(num => num % 2 === 0);
console.log(lastEvenIndex); // Output: 5
toReversed
The toReversed()
method is used to reverse the array without changing the original one.
Example:
const numbers = [1, 2, 3, 4, 5, 6];
const reversedNumbers = numbers.toReversed();
console.log(reversedNumbers); // Output: [6, 5, 4, 3, 2, 1]
console.log(numbers); // Output: [1, 2, 3, 4, 5, 6]
toSorted
The toSorted()
method is used for sorting an array without modifying the original array.
Example:
const numbers = [3, 1, 4, 1, 5, 9];
const sortedNumbers = numbers.toSorted();
console.log(sortedNumbers); // Output: [1, 1, 3, 4, 5, 9]
console.log(numbers); // Output: [3, 1, 4, 1, 5, 9]
with
The with()
method is used for updating an element in an array without modifying the original array.
Example:
const numbers = [1, 2, 3, 4, 5, 6];
const updatedNumbers = numbers.with(2, 99);
console.log(updatedNumbers); // Output: [1, 2, 99, 4, 5, 6]
console.log(numbers); // Output: [1, 2, 3, 4, 5, 6]
Follow Us:
Stay updated with our latest tips and tutorials by subscribing to our YouTube Channel.