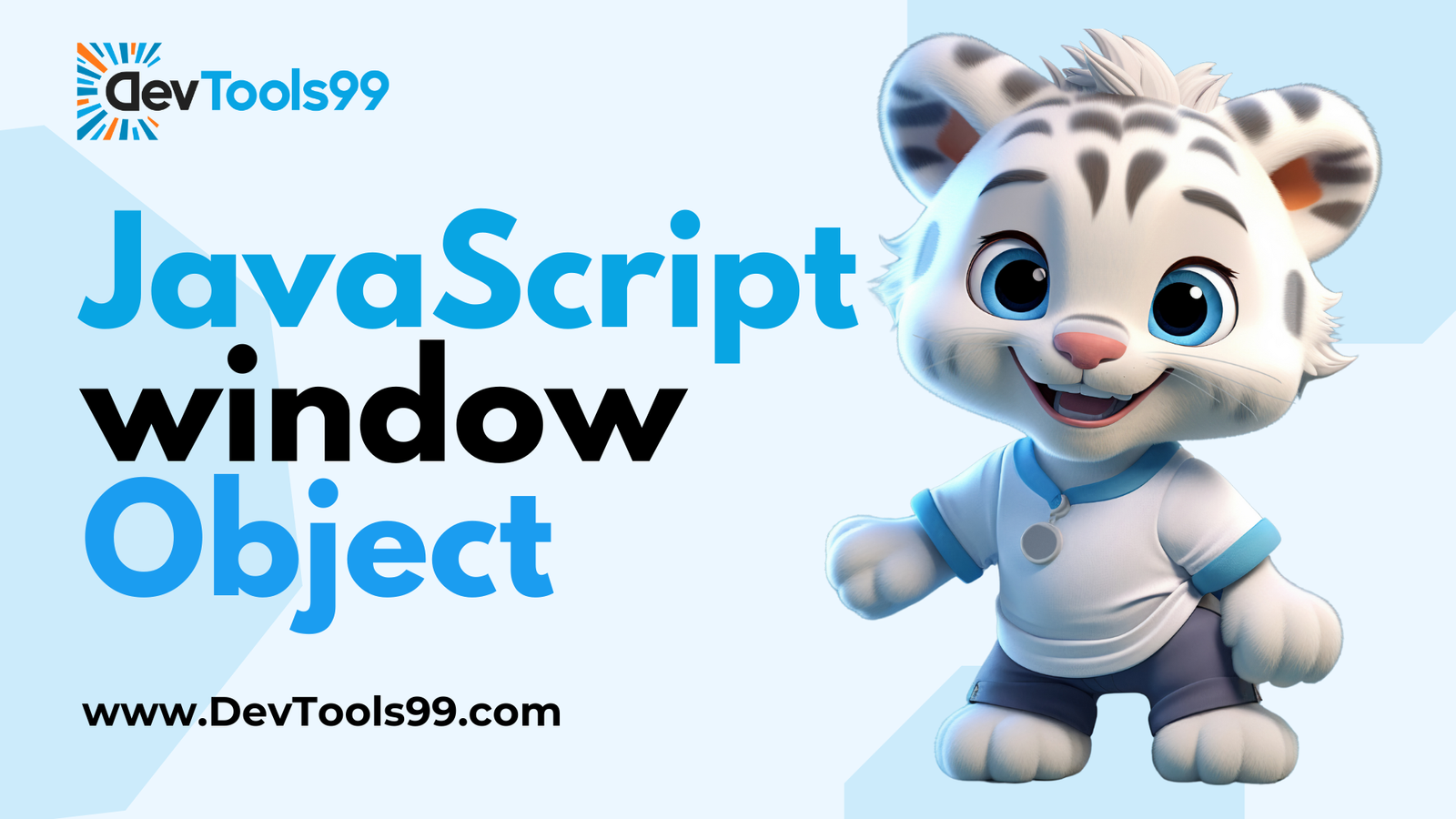
JavaScript window
Object
Introduction
In this tutorial, we will explore some of the most popular properties of the window
object, which is a representation of an open window in a browser. The window
object is the global object for JavaScript in web browsers, meaning all global JavaScript objects, functions, and variables automatically become members of the window
object.
Contents of Part 1:
Location
The location
property returns a Location
object, which contains information about the current URL of the window.
Example
// Reload the page after 2 seconds
setTimeout(() => {
window.location.reload();
}, 2000);
History
The history
property returns a History
object, which contains the pages visited by the user within the browser session.
Example
// Go back to the previous page after 2 seconds
setTimeout(() => {
window.history.go(-1);
}, 2000);
// Or
setTimeout(() => {
window.history.back();
}, 2000);
Document
The document
property returns the Document
object, which represents the HTML document loaded into the window.
Example
// Get the title of the page
console.log(window.document.title);
// Output: my app
// Change the body of the page
window.document.body.innerHTML = "hello world";
Navigator
The navigator
property returns a Navigator
object, which contains information about the browser.
Example
// Get the preferred language of the user
console.log(window.navigator.language);
// Output: en-US
// Get location coordinates
if (navigator.geolocation) {
window.navigator.geolocation.getCurrentPosition(pos => {
console.log(pos);
});
} else {
console.log("Couldn't get the position");
}
Screen
The screen
property returns a Screen
object, which contains information about the user's screen.
Example
// Get the height of the screen
console.log(window.screen.height);
// Output: 900
// Get the width of the screen
console.log(window.screen.width);
// Output: 1440
Remarks
When writing vanilla JavaScript code, you can use shortcuts like in the examples below. If you are working on a React app, for location
and history
you may want to use hooks provided by react-router-dom
.
Examples
// Using vanilla JavaScript
window.document.getElementById('root');
// Or
document.getElementById('root');
window.location.reload();
// Or
location.reload();
window.localStorage.setItem('name', 'Joe Doe');
// Or
localStorage.setItem('name', 'Joe Doe');
window.history.back();
// Or
history.back();
// In React
const history = useNavigate();
const location = useLocation();
Conclusion
The window
object is fundamental in JavaScript for interacting with the browser. Understanding its properties and methods allows developers to control and manipulate browser behavior effectively. This tutorial covered some key properties like location
, history
, document
, navigator
, and screen
. Mastering these will enhance your web development skills.
Follow Us:
Stay updated with our latest tips and tutorials by subscribing to our YouTube Channel.