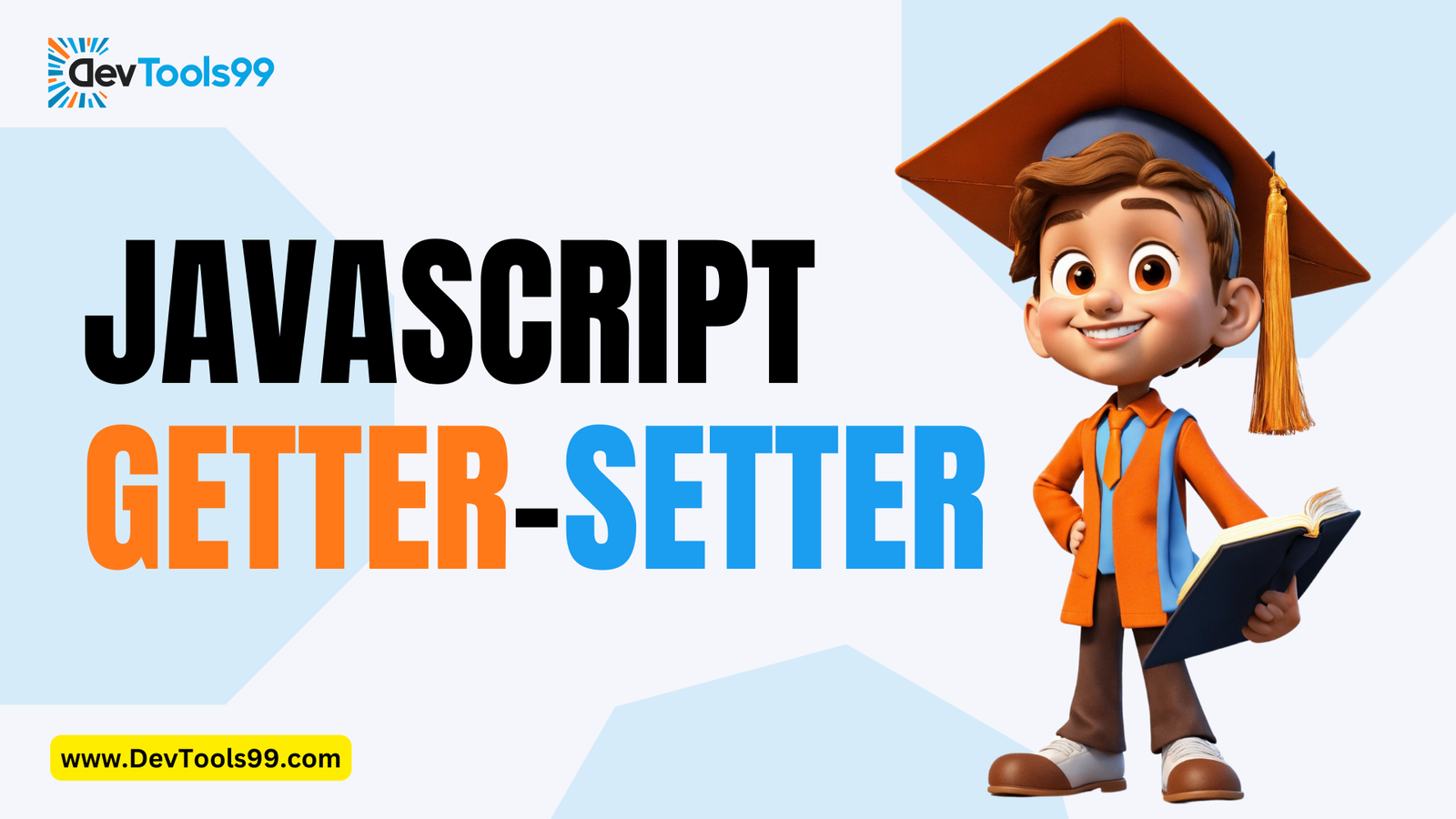
JavaScript Getter and Setter
Introduction
In JavaScript, getters and setters allow you to define object properties that behave like methods but are accessed like properties. They provide a way to intercept property access and assignment, adding custom logic before returning or setting a value. This can be particularly useful for validating data, performing calculations, or logging.
Data Property
A data property is a simple property that stores a value. It can be directly accessed and modified using the object.property
syntax.
const student = {
firstName: 'Monica'
};
console.log(student.firstName); // Monica
Accessor Property
Accessor properties are methods that allow you to define custom behavior for getting and setting the value of a property. They are defined using get
and set
keywords.
get
to define a getter method that returns the property value.set
to define a setter method that sets the property value.
JavaScript Getter
Getter methods are used to access the properties of an object. They allow you to define a method that will be executed when a property is accessed. The get
keyword is used to define a getter method.
const student = {
firstName: 'Monica',
get getName() {
return this.firstName;
}
};
console.log(student.getName); // Monica
// When you try to access the value as a method, an error occurs
// console.log(student.getName()); // error
In this example, the getter method getName
is created to access the firstName
property of the student
object. Note that the getter is accessed like a property, not like a method.
JavaScript Setter
Setter methods are used to change the values of an object. They allow you to define a method that will be executed when a property is assigned a value. The set
keyword is used to define a setter method.
const student = {
firstName: 'Imtiyaz',
set changeName(newName) {
this.firstName = newName;
}
};
console.log(student.firstName); // Imtiyaz
// Change (set) object property using a setter
student.changeName = 'Sarah';
console.log(student.firstName); // Sarah
In this example, the setter method changeName
is created to change the firstName
property of the student
object. The setter method is invoked when the property changeName
is assigned a new value.
Note that setter methods must have exactly one parameter, which is the value being set.
Object.defineProperty()
The Object.defineProperty()
method can also be used to add getters and setters to an object. This method allows more control over the property attributes.
const student = {
firstName: 'Imtiyaz'
};
// Adding a getter
Object.defineProperty(student, 'getName', {
get: function() {
return this.firstName;
}
});
// Adding a setter
Object.defineProperty(student, 'changeName', {
set: function(value) {
this.firstName = value;
}
});
console.log(student.firstName); // Imtiyaz
// Changing the property value
student.changeName = 'Sarah';
console.log(student.firstName); // Sarah
The Object.defineProperty()
method takes three arguments:
- The first argument is the object name.
- The second argument is the name of the property.
- The third argument is an object that describes the property, including
get
andset
functions.
Using Object.defineProperty()
, you can add or modify properties on an object with more precision, including setting attributes like configurable, enumerable, and writable.
Follow Us:
Stay updated with our latest tips and tutorials by subscribing to our YouTube Channel.