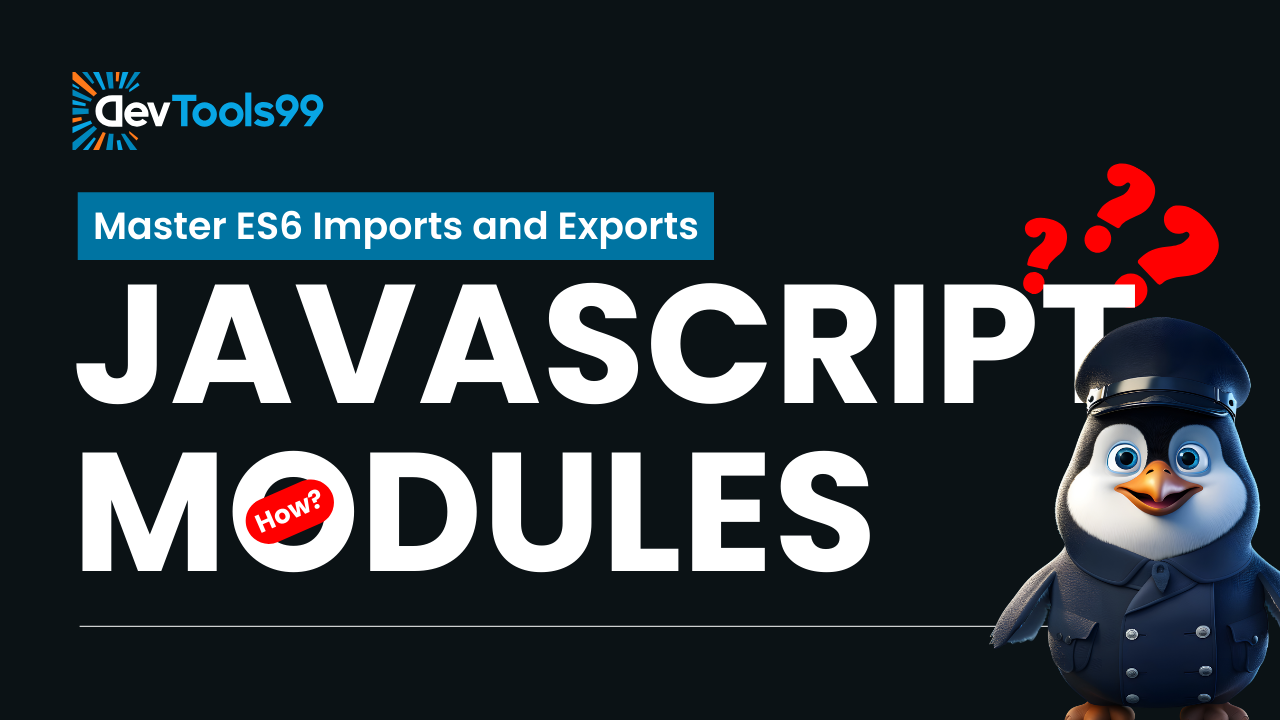
Understanding How JavaScript Modules Work: A Comprehensive Guide
JavaScript has evolved significantly in recent years, and one of the most important additions to the language is the ES6 modules system. This system provides a structured way to organize your code into smaller, reusable components, making it easier to manage, scale, and maintain large applications.
In this post, we'll dive into the key concepts of JavaScript modules, how they work, and how you can effectively use them to create cleaner, more maintainable codebases.
What Are JavaScript Modules?
Modules in JavaScript refer to independent, reusable pieces of code that can be imported into or exported from other files. This modular approach enhances readability, maintainability, and scalability, particularly in larger projects. Before the introduction of ES6, JavaScript did not have a built-in module system, and developers had to rely on third-party libraries (like CommonJS
or AMD
) to organize their code. With ES6, the import
and export
keywords allow for a more standardized and powerful approach to modularity.
Exporting and Importing Functions
The export
keyword allows you to expose functions, variables, or objects from a module so they can be used in other files. By exporting a function, for example, you enable its reuse without duplicating code, promoting better organization and maintainability.
Here's an example:
// utility.js
export function greet(name) {
return `Hello, ${name}!`;
}
// main.js
import { greet } from './utility.js';
console.log(greet('Alice')); // Output: Hello, Alice!
In this example, we exported the greet
function from the utility.js
file. In main.js
, we imported it using the import
statement and called it with the argument "Alice".
Default Exports
Sometimes, you may want to export a single item from a module as the default export. Default exports simplify the import process because you don't need to use curly braces when importing, and you can assign any name to the imported entity.
Here's how it works:
// math.js
export default function add(a, b) {
return a + b;
}
// main.js
import sum from './math.js';
console.log(sum(5, 3)); // Output: 8
In this example, the add
function is exported as the default export in math.js
. When importing it into main.js
, we can assign any name to it—in this case, sum
—and use it directly.
Named vs. Default Exports
There are two types of exports in JavaScript modules: named exports and default exports.
- Named exports allow you to export multiple entities from a single file, each with its own unique name.
- Default exports are used when you want to export a single entity as the primary export of a module.
Both export types can be used together in the same module. Here's an example:
// data.js
export const API_URL = 'https://api.example.com';
export default function fetchData(endpoint) {
return fetch(`${API_URL}/${endpoint}`);
}
// main.js
import fetchData, { API_URL } from './data.js';
console.log(API_URL); // Output: https://api.example.com
fetchData('users').then(console.log);
In this example, API_URL
is a named export, while the fetchData
function is exported as the default export. In main.js
, we imported both using a combination of default and named imports.
Importing Everything from a Module
Sometimes you might want to import all the exports from a module at once. This can be done using the *
syntax, which imports everything into a single object. Each export from the module will then be accessible as properties of that object.
Here's an example:
// constants.js
export const PI = 3.14;
export const E = 2.71;
// main.js
import * as constants from './constants.js';
console.log(constants.PI); // Output: 3.14
console.log(constants.E); // Output: 2.71
In this case, all the constants from constants.js
are imported into the constants
object, which can then be used to access individual values.
Re-exporting Modules
Re-exporting allows you to consolidate multiple exports from different modules into a single entry point. This helps to create cleaner and more organized code, particularly when you're building a library or an API that exposes functionality from several modules.
Here's an example of how to re-export modules:
// shapes.js
export * from './circle.js';
export * from './rectangle.js';
// main.js
import { drawCircle, drawRectangle } from './shapes.js';
drawCircle();
drawRectangle();
In this case, both circle.js
and rectangle.js
are re-exported through the shapes.js
module, allowing you to import them from a single source in main.js
.
Conclusion
ES6 modules are a cornerstone of modern JavaScript development. They enable better modularity, code reuse, and organization, making it easier to work on large codebases and collaborate with teams. Whether you’re building utilities, organizing components, or developing libraries, mastering import
and export
is essential for writing scalable and maintainable code.
Which module pattern do you find most useful in your projects? Have you used ES6 modules in your workflow? Let us know in the comments!
Follow Us:
Stay updated with our latest tips and tutorials by subscribing to our YouTube Channel.