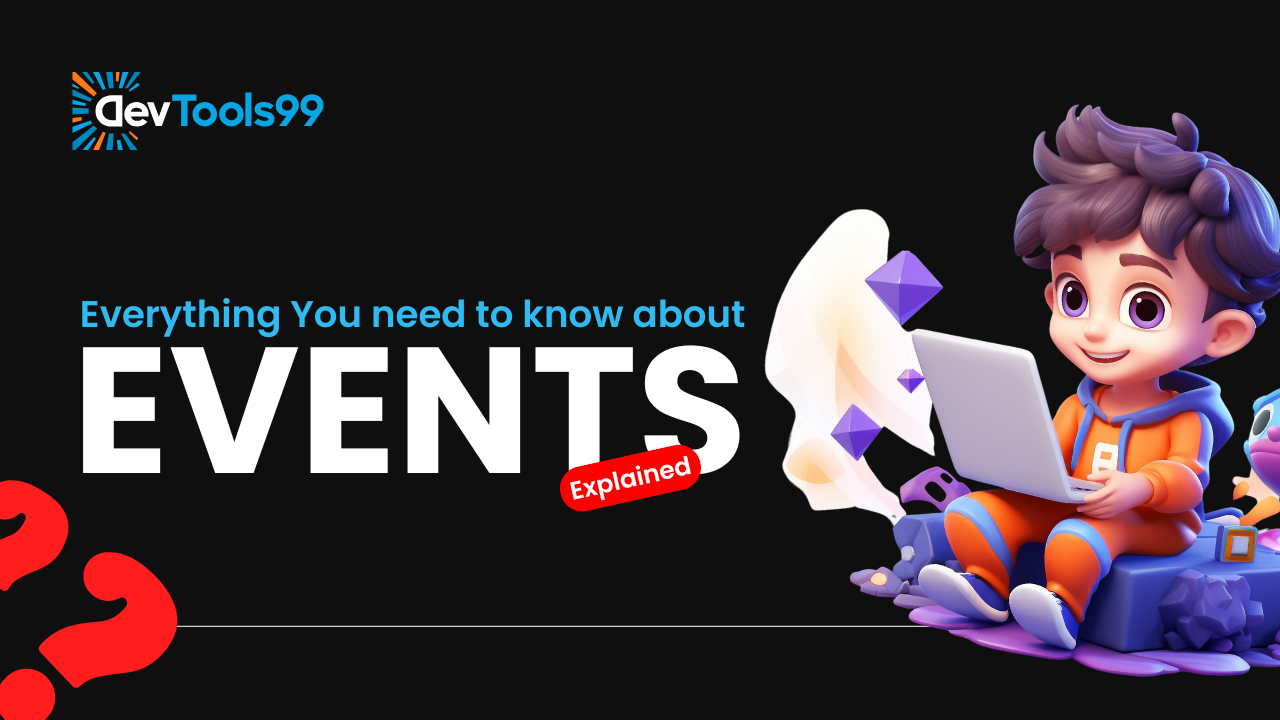
Everything You Need to Know About JavaScript Events
JavaScript events are an essential concept in web development, enabling web pages to respond to user actions like clicks, mouse movements, key presses, and more. These events play a crucial role in building dynamic and interactive web applications. In this blog, we'll dive deep into JavaScript events, explain how event handling works, discuss the differences between event bubbling and capturing, and offer practical examples to help you grasp the concept thoroughly.
What Are JavaScript Events?
An event is a signal or a notification triggered by an action or occurrence within the browser. Events are typically user-driven actions like clicking a button, submitting a form, or typing text in an input field. These events allow us to execute specific actions or functions when something happens on the webpage.
For example, a click
event is triggered when a user clicks on an element like a button. A mouseover
event is triggered when the mouse pointer hovers over an element. Understanding how events work is fundamental to creating interactive web applications.
Event Handlers and Event Listeners
In JavaScript, we respond to events using event handlers. An event handler is a function that gets executed when a specific event occurs on an element. To attach an event handler to an element, we use the addEventListener
method. This method allows you to listen for a specific event and execute a callback function when the event is triggered.
Syntax of addEventListener
target.addEventListener(event, function, useCapture);
- target: The HTML element that will listen for the event (e.g., a button or a div).
- event: The type of event to listen for, such as
'click'
,'keydown'
, or'mouseover'
. - function: The callback function that gets executed when the event is triggered. This function contains the logic you want to run in response to the event.
- useCapture (optional): A boolean value that determines the event propagation phase. If set to
true
, the event will be captured during the capturing phase. If set tofalse
(or omitted), the event will use the default bubbling phase.
Here's an example of how you might use addEventListener
:
document.querySelector('button').addEventListener('click', function() { alert('Button was clicked!'); });
In this example, when the user clicks the button, an alert will pop up with the message "Button was clicked!"
The DOM Tree and Event Propagation
JavaScript events do not happen in isolation; they travel through the Document Object Model (DOM) tree. The DOM represents the structure of the webpage as a tree of HTML elements, where each element is a node. Event propagation defines how events travel through this tree and how they are handled by different elements.
Events in JavaScript propagate in two primary phases: event bubbling and event capturing.
Understanding Event Propagation
Event propagation is the process by which an event moves through the DOM tree. When an event is triggered, it travels through the tree in a specific order. The two main phases are:
- Event Bubbling: In this phase, the event starts at the target element (the element that triggered the event) and bubbles up to its ancestors, including the parent, grandparent, and so on.
- Event Capturing: In contrast to bubbling, event capturing starts at the root ancestor of the DOM (the
<html>
element) and moves down the tree towards the target element.
Event Bubbling Explained
Event bubbling is the default behavior in most browsers. When an event is triggered, the event handler attached to the target element gets executed first, and then the event "bubbles" up the DOM, triggering event handlers on each ancestor element in turn.
Let's look at an example with three nested <div>
elements: grandparent, parent, and child. If we add event handlers to each of these elements:
- Click on the Child Div: The event starts at the child element and bubbles up to the parent and then the grandparent.
- Click on the Parent Div: The event starts at the parent and bubbles up to the grandparent, without triggering the child’s event handler.
This behavior can be useful for handling events on parent elements, as you can set up a single event listener on the parent to catch events from any of its child elements.
Event Capturing (Trickling) Explained
Event capturing (also known as trickling) is the opposite of bubbling. To enable event capturing, you need to set the useCapture
parameter to true
when attaching the event listener. In this phase, the event starts at the root ancestor (usually <html>
) and travels down the DOM tree towards the target element.
Consider the same three nested <div>
elements as before, but this time with useCapture = true
:
- Click on the Child Div: The event starts at the grandparent, moves to the parent, and finally reaches the child.
- Click on the Parent Div: The event starts at the grandparent and moves to the parent.
Event capturing is less commonly used but can be useful when you want to intercept events before they reach the target element.
Combining Bubbling and Capturing
In some cases, you may want to use both event capturing and event bubbling. If you attach event listeners with both useCapture = true
and useCapture = false
(the default), the event will first propagate through the capturing phase, then through the bubbling phase.
For example, if a parent element is set to capture an event, it will intercept the event during the capturing phase. Once the event has reached the target element, it will bubble up through the ancestors, triggering any bubbling event handlers.
Example of Mixed Capturing and Bubbling
Let's say you have a nested <div>
structure with a button inside the child. You want to handle the click event at both the capturing and bubbling phases:
document.querySelector('.grandparent').addEventListener('click', function() { alert('Capturing Phase: Grandparent'); }, true); // Capturing Phase document.querySelector('.parent').addEventListener('click', function() { alert('Bubbling Phase: Parent'); }); // Bubbling Phase document.querySelector('.child').addEventListener('click', function() { alert('Bubbling Phase: Child'); });
When the user clicks on the child element, the events will propagate as follows:
- The event will first be captured by the grandparent (capturing phase).
- Then, the event will bubble up to the parent and child elements (bubbling phase).
Summary
- Event Bubbling: The event starts at the target element and bubbles up to its ancestors in the DOM tree.
- Event Capturing: The event starts at the root ancestor of the DOM tree and travels down towards the target element.
- Combining Both Phases: If both bubbling and capturing are used, the event will first go through the capturing phase, followed by the bubbling phase.
Understanding the difference between event bubbling and capturing, and knowing how to control event propagation, gives you greater flexibility and control over how events are handled in your web applications. This knowledge will help you create more interactive and dynamic user interfaces.
Follow Us:
Stay updated with our latest tips and tutorials by subscribing to our YouTube Channel.