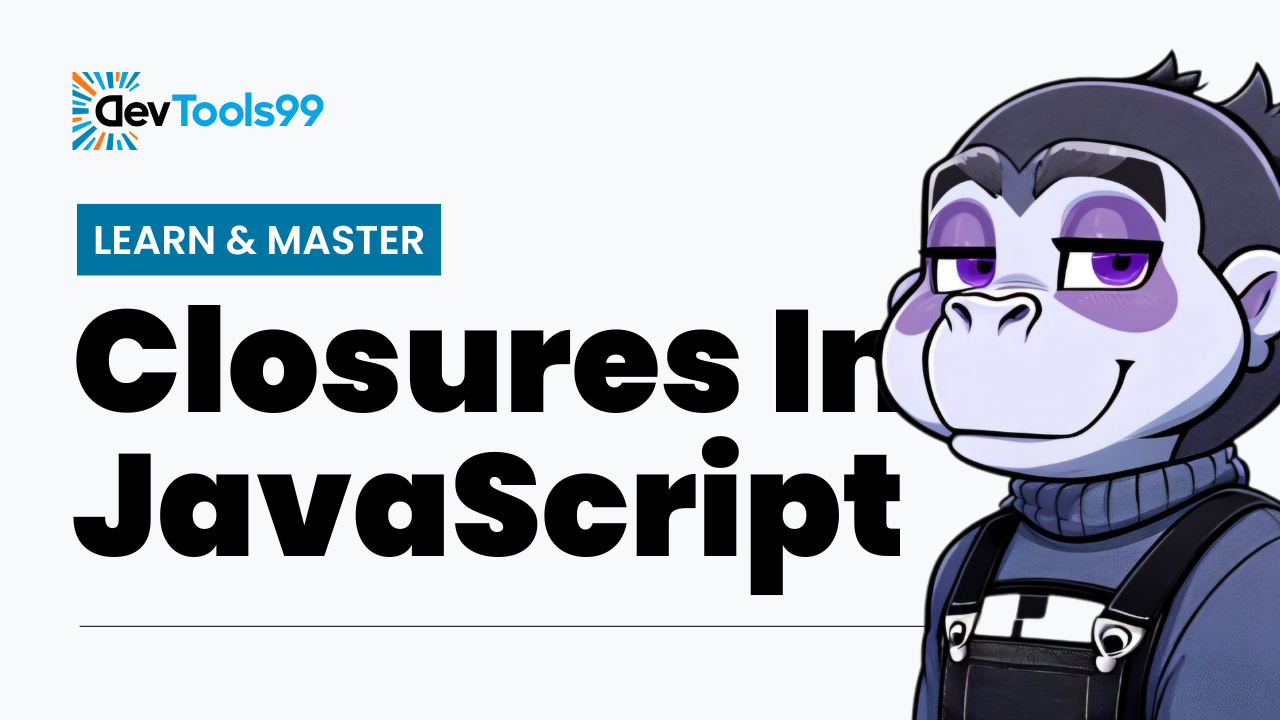
Learn & Master Closures In JavaScript
JavaScript closures are a powerful feature that every developer should understand to write cleaner, more efficient code. Closures allow functions to retain access to their outer scope even after execution, making them useful in various scenarios like data privacy, function factories, and event handling.
What is a JavaScript Closure?
A closure in JavaScript is created when a function is defined inside another function, and the inner function retains access to the outer function’s variables even after the outer function has executed.
function outerFunction() {
let outerVariable = "I'm from the outer function";
function innerFunction() {
console.log(outerVariable);
}
return innerFunction;
}
const closureExample = outerFunction();
closureExample(); // Outputs: "I'm from the outer function"
Even though outerFunction
has completed execution, innerFunction
retains access to outerVariable
due to the closure.
Key Use Cases of Closures
1) Data Privacy
Closures are commonly used to create private variables that cannot be accessed from outside the function.
function counter() {
let count = 0;
return {
increment: function() {
count++;
console.log(count);
},
decrement: function() {
count--;
console.log(count);
}
};
}
const myCounter = counter();
myCounter.increment(); // 1
myCounter.increment(); // 2
myCounter.decrement(); // 1
Here, count
is private and can only be modified via increment
and decrement
.
2) Function Factories
Closures are great for creating functions dynamically.
function greetingFactory(greeting) {
return function(name) {
console.log(`${greeting}, ${name}!`);
};
}
const sayHello = greetingFactory("Hello");
sayHello("Alice"); // Outputs: "Hello, Alice!"
The function returned by greetingFactory
retains access to greeting
even after execution.
3) Event Handlers in Loops
Closures help ensure event handlers capture the correct value in loops.
for (let i = 1; i <= 3; i++) {
setTimeout(function() {
console.log(i);
}, 1000 * i);
}
This ensures each setTimeout
callback captures the correct value of i
instead of all logging the final value.
Understanding Closures with Practical Examples
Closures play a vital role in optimizing performance and handling asynchronous code efficiently. They appear in many everyday coding scenarios, including:
- Memoization: Caching results to optimize function performance.
- Currying: Transforming functions into a series of functions that accept one argument at a time.
- Managing Asynchronous Code: Keeping track of state even after an async operation is delayed.
Memoization Example
Memoization is a technique that stores expensive function call results and returns cached values when the same inputs occur.
function memoize(fn) {
let cache = {};
return function(arg) {
if (cache[arg]) {
return cache[arg];
}
let result = fn(arg);
cache[arg] = result;
return result;
};
}
function square(num) {
return num * num;
}
const memoizedSquare = memoize(square);
console.log(memoizedSquare(4)); // 16 (calculated)
console.log(memoizedSquare(4)); // 16 (cached)
Conclusion
JavaScript closures are an essential concept for writing clean and efficient code. They enable data encapsulation, help in creating dynamic functions, and play a crucial role in handling asynchronous behavior. By mastering closures, you'll gain a deeper understanding of JavaScript’s behavior and unlock new possibilities for structuring your applications. Start practicing with closures today and see how they can improve your code!
Follow Us:
Stay updated with our latest tips and tutorials by subscribing to our YouTube Channel