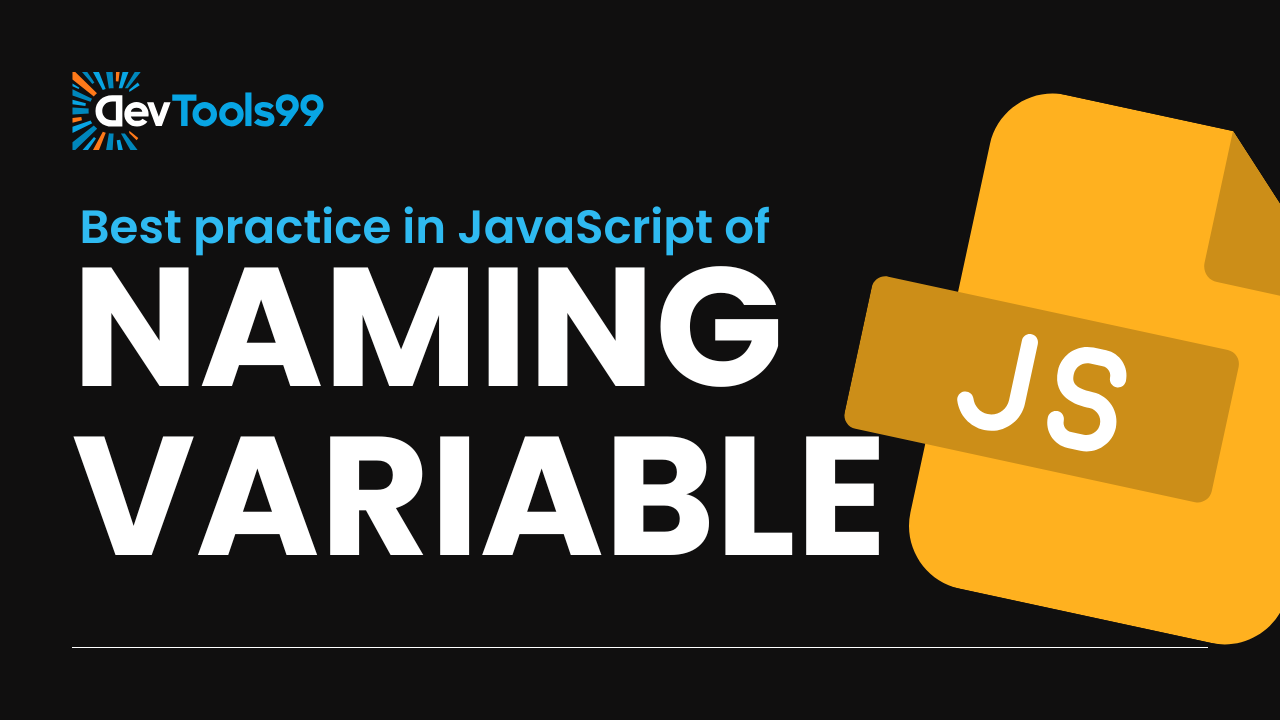
Mastering Variable Naming in JavaScript: Best Practices
Improve your JavaScript code with these variable naming tips for clarity, consistency, and maintainability.
Introduction
Variable naming is a crucial aspect of writing clean and maintainable JavaScript code. Properly named variables make your code easier to understand and debug, saving time and effort for you and your team. In this blog, we’ll explore best practices for naming variables in JavaScript, ensuring clarity, consistency, and readability in your code.
1. Clarity Over Brevity
Always prioritize clarity over brevity when naming variables. Descriptive names that clearly explain the purpose of the variable are far more valuable than short, cryptic names. For instance:
let userAge = 25; // Clear and descriptive
Avoid ambiguous names like let x = 25;
, as they do not convey the variable's purpose.
2. Use Camel Case
JavaScript follows camel case convention for naming variables. This means the first word is in lowercase, and subsequent words are capitalized. For example:
let firstName = "John"; // Camel case
Consistent use of camel case ensures better readability across your codebase.
3. Maintain Consistency
Consistency is key to readable and maintainable code. If you start with a naming convention, stick to it. For example, if you use userName
, avoid switching to user_name
in the same codebase.
4. Avoid Single-Letter Variables
While single-letter variables like x
or y
might seem convenient, they are rarely descriptive. Instead, use meaningful names:
let totalPrice = 100; // Descriptive
5. Reflect Purpose in Names
Variable names should clearly reflect their purpose. For example:
let itemsInCart = 5; // Reflects what the variable represents
6. Avoid Magic Numbers
Magic numbers are hard-coded values that lack context. Replace them with constants for better readability and maintainability:
const MAX_USERS = 10; // Replaces the magic number 10
7. Provide Context in Names
Including context in your variable names makes them more intuitive. For example:
let isAuthenticated = false; // Provides clear context
8. Avoid Reserved Keywords
Never use reserved keywords as variable names. For instance:
let userName = "Alice"; // Correct
// let function = "test"; // Incorrect
9. Boolean Variables: Use Prefixes
For boolean variables, use prefixes like is
or has
to indicate their purpose:
let hasPermission = true; // Clear and readable
10. Limit Variable Scope
Declare variables in the narrowest scope possible to avoid polluting the global namespace and improve code clarity. For instance:
function calculateTotal() {
let totalAmount = 0; // Limited to the function's scope
}
Conclusion
By following these best practices for naming variables in JavaScript, you can write code that is easier to read, understand, and maintain. Clear, consistent, and descriptive variable names make a significant difference in your programming workflow. Start implementing these tips in your projects today, and happy coding!
Follow Us:
Stay updated with our latest tips and tutorials by subscribing to our YouTube Channel.