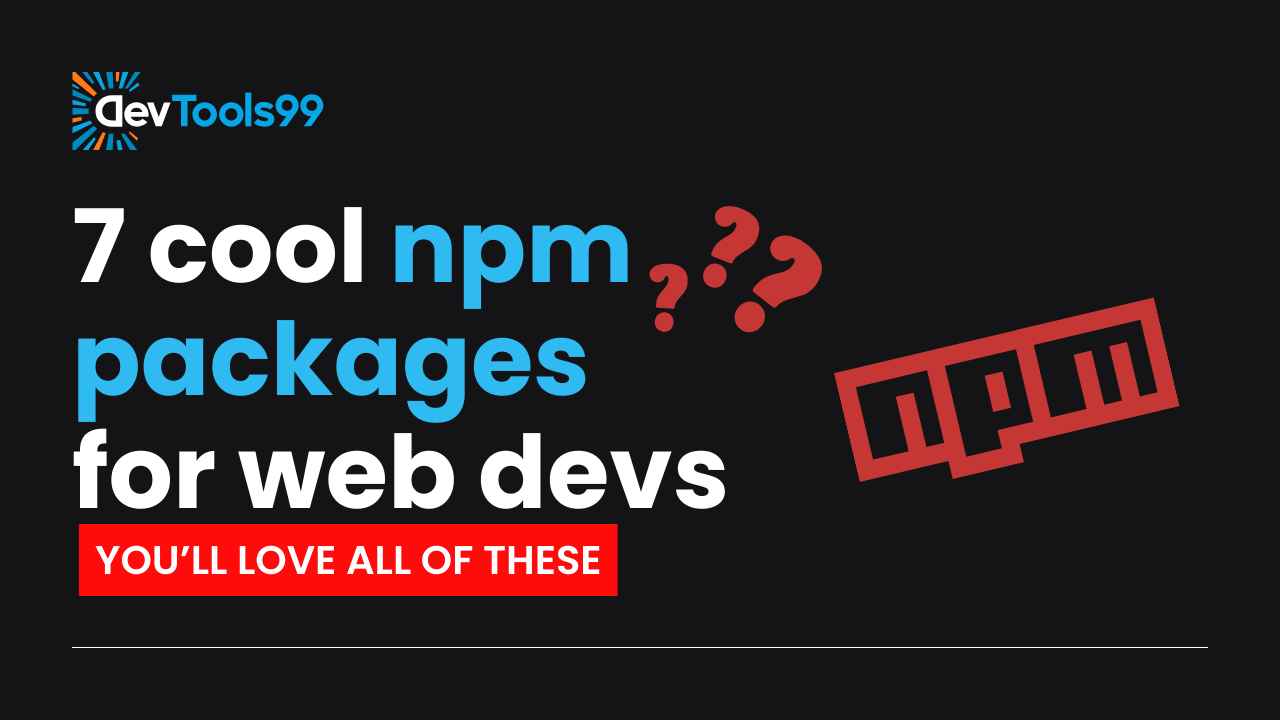
7 Cool NPM Packages for Web Developers
In the dynamic realm of web development, npm (Node Package Manager) serves as a crucial gateway to a myriad of libraries and modules, supercharging projects with enhanced functionality. Today, we delve into seven npm packages that promise to elevate your productivity and streamline your workflow in web development.
1. Lodash
Lodash is a comprehensive utility library designed to facilitate easier manipulation of JavaScript objects, arrays, strings, and numbers. It excels in tasks such as deep cloning, merging arrays, and more, providing developers with a robust set of tools that promote code efficiency and simplicity.
const _ = require('lodash');
const array = [1, 2, 3];
const newArray = _.reverse(array);
console.log(newArray); // [3, 2, 1]
Lodash streamlines complex data operations like sorting, filtering, and transforming, making it an invaluable asset in any JavaScript-centric development environment.
2. Async
Async is a versatile library that enhances the management of asynchronous operations in JavaScript. It is particularly useful for coordinating multiple asynchronous tasks such as API requests, seamlessly handling complex workflows with its series, parallel, and concurrency controls.
const async = require('async');
async.parallel([
function(callback) {
setTimeout(() => {
console.log('First Task');
callback(null, 'one');
}, 200);
},
function(callback) {
setTimeout(() => {
console.log('Second Task');
callback(null, 'two');
}, 100);
}
], function(err, results) {
console.log(results); // ['one', 'two']
});
Async simplifies handling of callbacks, promises, and async/await, enhancing code readability and reducing complexity in large-scale projects.
3. Chalk
Chalk enhances developer experiences by allowing colorful styling of terminal outputs. This feature is crucial for distinguishing between logs, warnings, and errors, improving the debug process significantly.
const chalk = require('chalk');
console.log(chalk.blue('Hello world!'));
console.log(chalk.red.bold('Error message'));
Chalk’s customization options facilitate improved visual management of console outputs, which is essential during development and debugging phases.
4. Dotenv
Dotenv is a pivotal package for managing environment variables within Node.js projects. It securely handles sensitive data like API keys and database credentials by externalizing them in a .env file, safeguarding your codebase against potential security risks.
require('dotenv').config();
console.log(process.env.SECRET_KEY);
By loading environment-specific settings at runtime, Dotenv enhances both the security and flexibility of applications across different deployment environments.
5. Express
Express stands as the quintessential framework for creating efficient web applications and APIs with Node.js. Its lightweight structure facilitates rapid server setup and route management, accommodating everything from simple web services to complex RESTful APIs.
const express = require('express');
const app = express();
app.get('/', (req, res) => {
res.send('Hello World!');
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
Express’s modularity and ease of use make it an ideal choice for developers looking to implement robust solutions swiftly.
6. Commander
Commander simplifies the creation of command-line interfaces in Node.js, offering a structured framework for defining commands and options. This tool greatly enhances the usability and maintainability of CLI applications by automating argument parsing and help text generation.
const { program } = require('commander');
program
.version('1.0.0')
.option('-n, --name <type>', 'Your name')
.parse(process.argv);
console.log(`Hello, ${program.name}!`);
For developers building custom tools and utilities, Commander provides a clear and effective way to manage command-line options.
7. Moment
Moment is renowned for its comprehensive handling of dates and times in JavaScript. Although it has entered maintenance mode, its functionality for formatting, parsing, and manipulating dates remains unmatched, especially for legacy projects.
const moment = require('moment');
console.log(moment().format('MMMM Do YYYY, h:mm:ss a')); // October 24th 2024, 12:00:00 pm
Moment's extensive locale support and intuitive API make it a dependable choice for projects involving complex date and time manipulation.
Conclusion
These seven npm packages—Lodash, Async, Chalk, Dotenv, Express, Commander, and Moment—are fundamental tools for any web developer aiming to enhance efficiency and productivity. Each package offers unique utilities that cater to various aspects of web application development, ensuring your projects run smoothly and efficiently.
Follow Us:
Stay updated with our latest tips and tutorials by subscribing to our YouTube Channel.