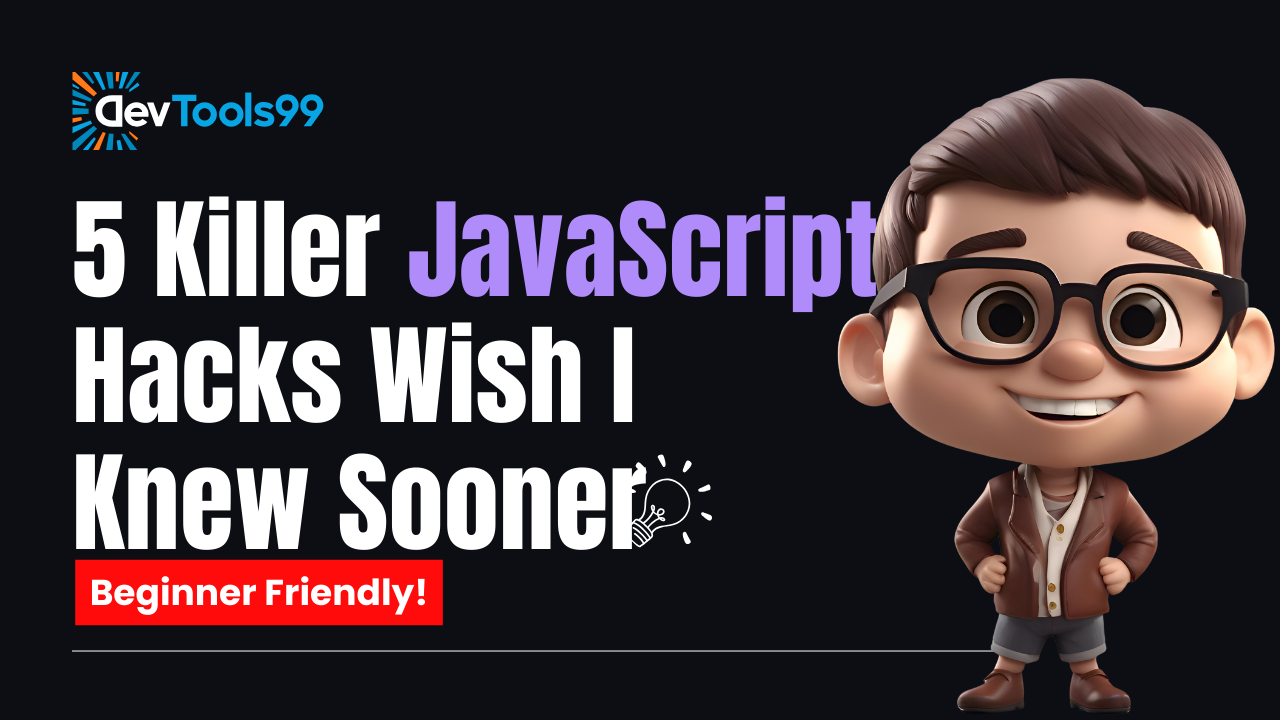
5 Killer JavaScript Hacks I Wish I Knew Sooner
JavaScript is a versatile and powerful language, but it's easy to overlook some of its most efficient tricks when you're caught up in the basics. Below are five JavaScript hacks that can make your code more concise, readable, and efficient. These are small but mighty techniques that will level up your coding game.
1. Short-Circuit Evaluation for Default Values
Instead of writing lengthy if-else
conditions to assign default values, you can use short-circuit evaluation with the ||
(logical OR) operator for a cleaner, more concise solution. This operator evaluates the left-hand side, and if it’s falsy (like null
, undefined
, or 0
), it assigns the default value on the right-hand side.
// Traditional approach
let value = inputValue;
if (!value) {
value = 'default';
}
// Short-circuit evaluation
let value = inputValue || 'default';
Short-circuit evaluation works because the ||
operator returns the first truthy value it finds. If the left-hand value is falsy, it moves on to the default value. This can make your code much more succinct and readable.
2. Swap Variables without a Temporary Variable
Swapping variables used to require a temporary variable to hold one of the values, but with array destructuring in modern JavaScript, you can swap variables in a single line. This not only makes your code more readable but also avoids the overhead of creating unnecessary temporary variables.
// Traditional approach
let a = 1, b = 2;
let temp = a;
a = b;
b = temp;
// Array destructuring approach
let a = 1, b = 2;
[a, b] = [b, a];
This trick is especially useful when dealing with multiple swaps in complex code, making your logic more intuitive and less error-prone.
3. Clone an Array Quickly
Cloning arrays is a common task in JavaScript, and it used to be done using methods like slice()
or looping through the array. But with the spread operator (...
), you can clone arrays effortlessly in a single line of code.
// Traditional approach
let original = [1, 2, 3];
let copy = original.slice();
// Spread operator approach
let original = [1, 2, 3];
let copy = [...original];
The spread operator is not only faster and more concise but also improves the readability of your code, making it clear that you're creating a shallow copy of the array.
4. Easily Remove Duplicates from an Array
Removing duplicates from an array used to be a tedious task involving loops or complex logic. With the introduction of Set
in JavaScript, you can easily filter out duplicates in a one-liner. A Set
only stores unique values, so it automatically removes duplicates.
// Traditional approach
let array = [1, 2, 2, 3, 4, 4];
let uniqueArray = array.filter((item, index) => array.indexOf(item) === index);
// Set approach
let array = [1, 2, 2, 3, 4, 4];
let uniqueArray = [...new Set(array)];
This approach simplifies the task of handling arrays with duplicate entries, providing a more efficient and elegant solution.
5. Convert a String to a Number Quickly
In JavaScript, you can convert a string to a number using parseInt()
or Number()
, but there's an even faster way: the unary +
operator. It’s a simple, one-character solution that gets the job done quickly and efficiently.
// Traditional approach
let num = parseInt("123");
let num = Number("123");
// Unary + approach
let num = +"123";
This method is perfect for quick conversions and can save time when you’re dealing with simple number strings. Just be aware that it will return NaN
for non-numeric strings.
Conclusion
These JavaScript hacks are just the tip of the iceberg when it comes to writing more efficient and elegant code. By incorporating these simple tricks into your workflow, you can streamline your code and save time on common tasks. Whether you're swapping variables or removing duplicates, these techniques will help you write better JavaScript with less effort.
Follow Us:
Stay updated with our latest tips and tutorials by subscribing to our YouTube Channel.