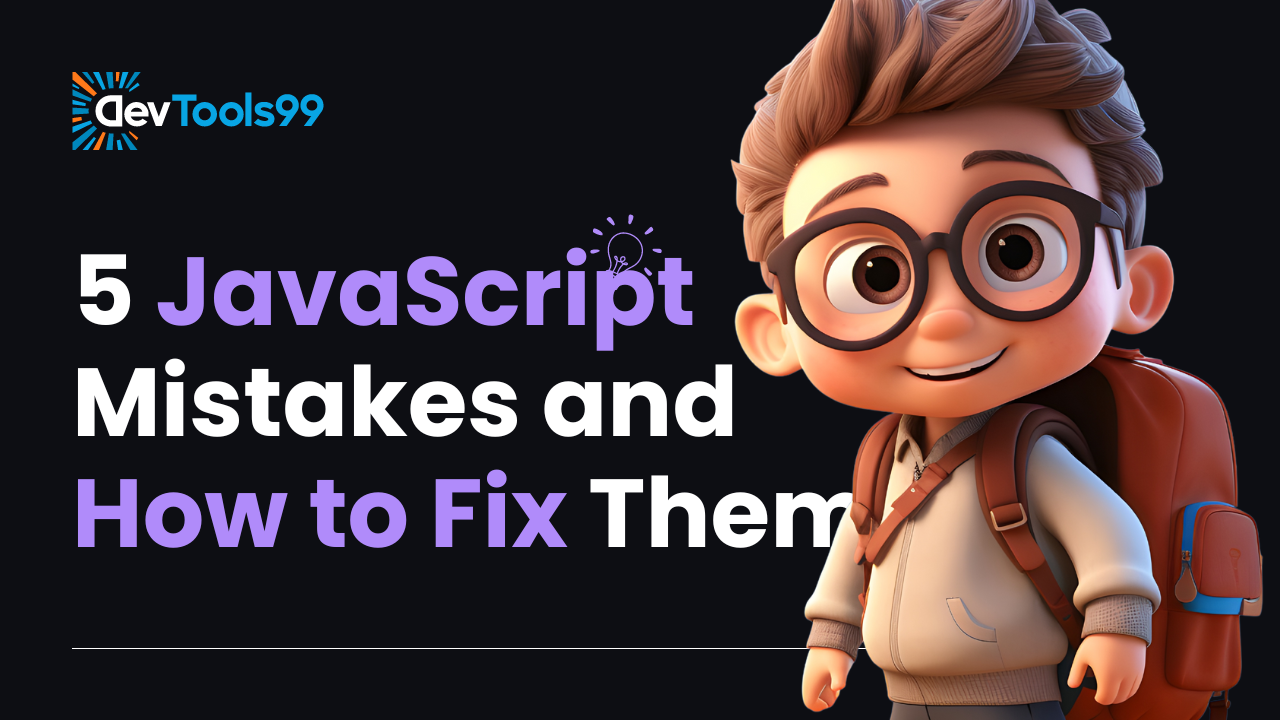
5 JavaScript Mistakes and How to Fix Them
JavaScript is a powerful and flexible programming language, but it's easy to fall into common traps that can lead to bugs and difficult-to-maintain code. In this post, we'll explore five common JavaScript mistakes and how to fix them, helping you write cleaner and more efficient code.
1. Forgetting to Declare Variables with let
or const
Omitting let
or const
when declaring variables can lead to the creation of global variables. This often results in unexpected behavior because these variables are not scoped to the block, function, or module, making them accessible everywhere, even when not intended.
How to Fix It
Always declare variables using let
for block-scoped variables that may change, and const
for variables that should remain constant.
// Example of incorrect code:
x = 10; // Global variable
// Correct code:
let x = 10; // Scoped variable
2. Ignoring async/await
for Asynchronous Code
Writing asynchronous code with nested callbacks (often called "callback hell") makes the code difficult to read and maintain. Instead of using callbacks, JavaScript provides the async/await
syntax, which simplifies working with promises and makes your code more readable.
How to Fix It
Refactor your asynchronous code to use async
and await
for handling promises. This will not only make your code cleaner but also easier to debug.
// Example of callback hell:
function fetchData(callback) {
fetch('url', response => {
process(response, result => {
save(result, () => {
console.log('Done');
});
});
});
}
// Using async/await:
async function fetchData() {
const response = await fetch('url');
const result = await process(response);
await save(result);
console.log('Done');
}
3. Not Using const
for Real Constant Values
Using let
for values that don’t change can lead to accidental reassignment, potentially causing bugs in your application. If a variable's value should remain constant throughout your code, always use const
.
How to Fix It
Use const
for variables that should not be reassigned after their initial declaration. This helps prevent bugs caused by unintended changes to critical values.
// Incorrect:
let PI = 3.14;
PI = 3.14159; // Accidental reassignment
// Correct:
const PI = 3.14;
// PI cannot be reassigned
4. Neglecting Error Handling
Failing to handle errors properly can cause your application to crash or behave unpredictably. In JavaScript, unhandled errors in asynchronous code, like promise rejections, can lead to silent failures.
How to Fix It
Always use proper error handling techniques such as try...catch
blocks or handling promise rejections with .catch()
to ensure your code runs reliably even when something goes wrong.
// No error handling:
async function getData() {
const response = await fetch('url');
const data = await response.json();
}
// With error handling:
async function getData() {
try {
const response = await fetch('url');
const data = await response.json();
} catch (error) {
console.error('An error occurred:', error);
}
}
5. Forgetting to Make Use of Object.freeze()
When dealing with objects that should not be altered, it's common to accidentally modify them, introducing bugs. Using Object.freeze()
prevents any changes to the object, ensuring immutability.
How to Fix It
Use Object.freeze()
on objects that should remain unchanged. This will protect them from accidental modifications, preserving the integrity of your data.
// Without Object.freeze():
const config = { theme: 'dark' };
config.theme = 'light'; // Accidental change
// With Object.freeze():
const config = Object.freeze({ theme: 'dark' });
config.theme = 'light'; // Error: Cannot modify a frozen object
Conclusion
Avoiding these common JavaScript mistakes can drastically improve the quality of your code. By properly declaring variables, using async/await
, leveraging const
for constants, handling errors, and freezing objects when necessary, you can create more stable and maintainable applications. Taking the time to address these issues now will save you a lot of debugging headaches in the future.
Follow Us:
Stay updated with our latest tips and tutorials by subscribing to our YouTube Channel.