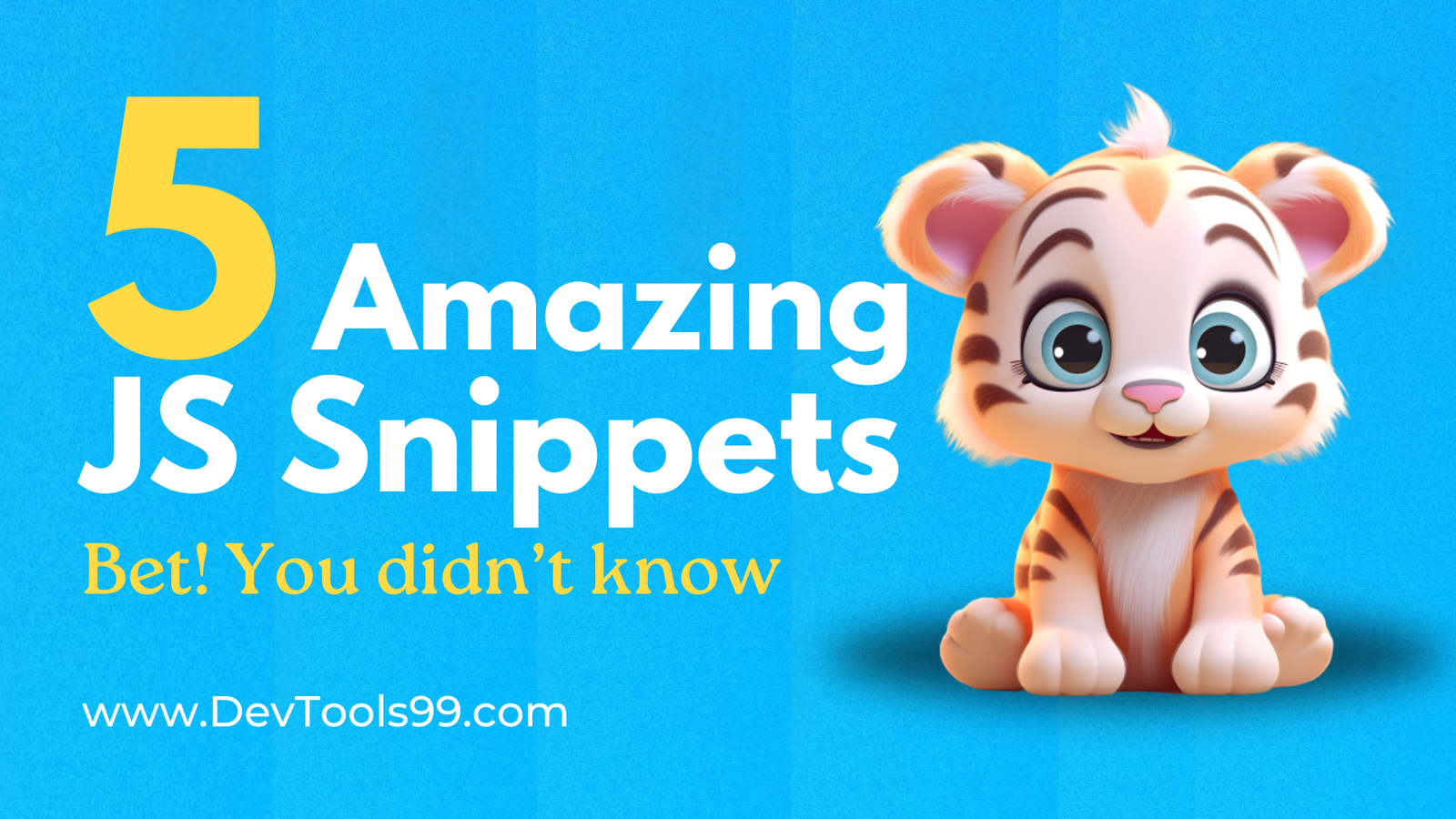
5 Amazing JavaScript Snippets Every Developer Should Know
Hey Devs! Whether you're a seasoned coder or just starting out, having a collection of handy JavaScript snippets can significantly speed up your workflow. In this post, we’re sharing five powerful JavaScript snippets that can help you achieve common tasks with ease. From triggering device vibrations to detecting dark mode, these snippets are sure to come in handy. Let’s dive into these practical examples!
1. Vibrate Your Device
Did you know that you can make your device vibrate using JavaScript? This can be particularly useful in creating custom notifications or feedback mechanisms in mobile web applications. You just need to specify the duration of the vibration in milliseconds.
// Vibrate the device for 300 milliseconds
window.navigator.vibrate(300);
Note: This feature might not work if the device is in silent mode.
2. Call a Function When Enter is Pressed
Sometimes you might want to trigger a function when the Enter key is pressed, such as when a user submits a form or finishes typing in a text area. This snippet allows you to do just that. By adding an event listener to the text area, you can detect when the Enter key is pressed and trigger the desired function.
let textArea = document.getElementById("ingstone");
textArea.addEventListener("keydown", function(e) {
if (e.key === "Enter") {
validate(e);
}
});
function validate() {
alert('Enter key is pressed');
}
3. Identify OS
If you need to detect the operating system of the user's device, this JavaScript snippet will do the trick. It checks the user's browser information and identifies the OS based on common patterns.
var osName = "Unknown OS";
if (navigator.appVersion.indexOf("Win") != -1) osName = "Windows";
if (navigator.appVersion.indexOf("Mac") != -1) osName = "iOS - Apple Inc.";
if (navigator.appVersion.indexOf("X11") != -1) osName = "UNIX";
if (navigator.appVersion.indexOf("Linux") != -1) osName = "Linux";
console.log(osName);
4. Copy to Clipboard
Copying text to the clipboard is a common task in web development. With this simple one-liner, you can copy any text to the clipboard. Just replace targetText
with the string you want to copy, and you're all set.
function copyToClipboard() {
navigator.clipboard.writeText(targetText);
}
5. Identify Whether Dark Mode is On or Off
As dark mode becomes increasingly popular, you may want to customize your website's appearance based on the user's preference. This snippet allows you to detect whether dark mode is enabled on the user's device and respond accordingly.
if (window.matchMedia && window.matchMedia('(prefers-color-scheme: dark)').matches) {
console.log('Dark Mode is enabled');
} else {
console.log('Light Mode is enabled');
}
These snippets are simple yet powerful tools that can enhance the functionality of your web applications. By incorporating them into your projects, you can save time and provide a better user experience. Try them out and see how they can elevate your coding game!
Happy coding!
Follow Us:
Stay updated with our latest tips and tutorials by subscribing to our YouTube Channel.