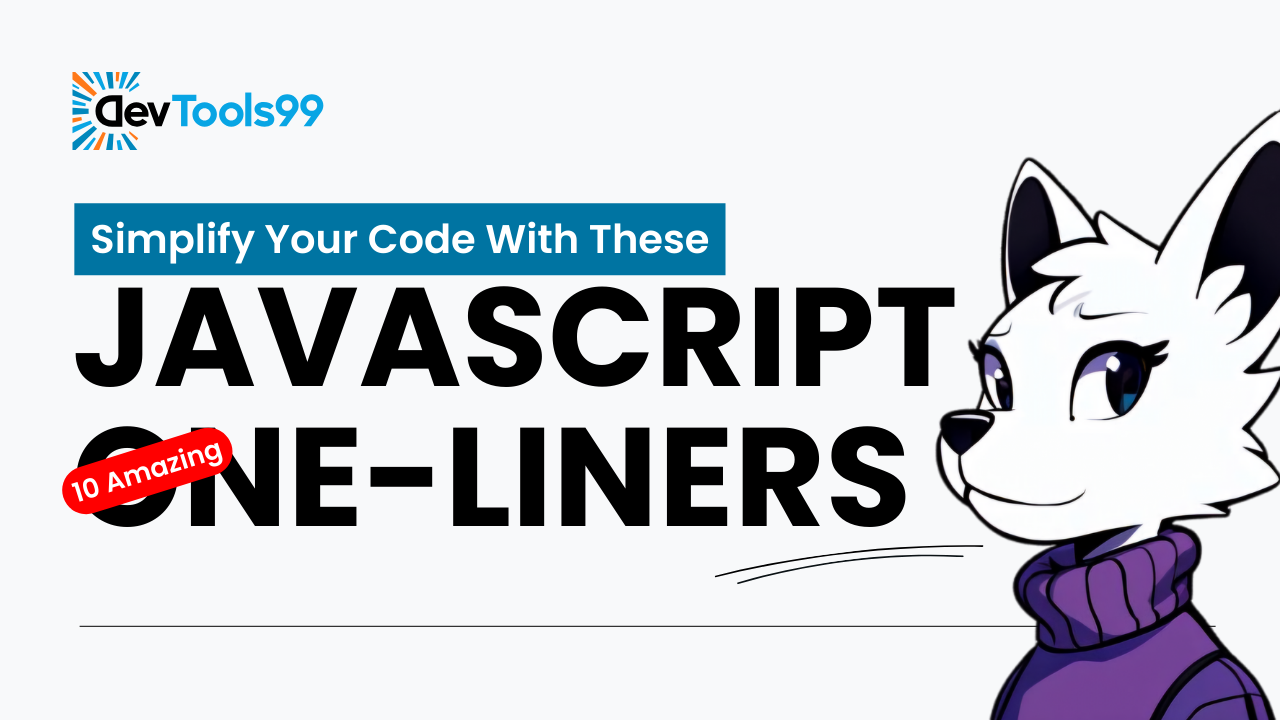
JavaScript One-Liners to Simplify Your Code
JavaScript is not only powerful but also flexible, allowing developers to achieve more with less code. One-liners are a clever way to reduce complexity in daily programming tasks. This article delves into 10 useful JavaScript one-liners, providing a detailed explanation of how each works to help you incorporate them seamlessly into your projects.
1. Remove Duplicates from an Array
const uniqueArray = [...new Set(array)];
The Set
object is a collection of unique values. When you convert an array into a Set
, duplicates are automatically removed. By using the spread operator (...)
, you can then expand this Set
back into an array. This approach is both succinct and efficient for ensuring all elements are unique.
Example:
const array = [1, 2, 2, 3, 4, 4, 5];
const uniqueArray = [...new Set(array)];
console.log(uniqueArray); // Outputs: [1, 2, 3, 4, 5]
2. Swap Two Variables Without a Temporary Variable
[a, b] = [b, a];
This technique leverages array destructuring to swap the values of two variables. It removes the need for a temporary variable and makes the swap intuitive and error-free by using a destructuring assignment.
Example:
let a = 1, b = 2;
[a, b] = [b, a];
console.log(a, b); // Outputs: 2, 1
3. Generate a Random Number Between a Range
const random = (min, max) => Math.random() * (max - min) + min;
This function utilizes Math.random()
to generate a random number and scales it to the desired range by multiplying with the difference between max and min, then adding the min. This ensures the result falls within the specified range.
Example:
console.log(random(5, 10)); // Outputs a random number between 5 and 10
4. Flatten a Multi-dimensional Array
const flatArray = array.flat(Infinity);
Multi-dimensional arrays can be simplified into a single-dimensional array using the flat()
method. The Infinity
parameter instructs the method to flatten all nested arrays regardless of their depth.
Example:
const array = [1, [2, [3, [4]]]];
const flatArray = array.flat(Infinity);
console.log(flatArray); // Outputs: [1, 2, 3, 4]
5. Short-Circuit Default Value
const value = someVariable || "Default Value";
This line employs the logical OR operator to provide a default value for a variable. If someVariable
is falsy (i.e., null
, undefined
, false
, 0
, ""
, or NaN
), "Default Value" is used instead.
Example:
const someVariable = null;
const value = someVariable || "Default Value";
console.log(value); // Outputs: "Default Value"
6. Shuffle an Array
const shuffled = array.sort(() => Math.random() - 0.5);
Although using sort()
with Math.random()
is a common technique for shuffling, it's worth noting that it does not provide a perfectly uniform shuffle. However, it is sufficient for many non-critical applications.
Example:
const array = [1, 2, 3, 4, 5];
const shuffled = array.sort(() => Math.random() - 0.5);
console.log(shuffled); // Outputs a shuffled version of the array
7. Get the Last Element of an Array
const lastElement = array.slice(-1)[0];
By using slice()
with a negative index, you can extract elements starting from the end of the array. This is particularly useful for accessing the last element directly without altering the original array.
Example:
const array = [1, 2, 3, 4];
const lastElement = array.slice(-1)[0];
console.log(lastElement); // Outputs: 4
8. Convert a Number to a String
const numString = number + '';
Concatenating a number with an empty string triggers JavaScript's type coercion, turning the number into a string. This method is quick and avoids more verbose methods like toString()
.
Example:
const number = 123;
const numString = number + '';
console.log(numString); // Outputs: "123"
9. Capitalize the First Letter of a String
const capitalized = str[0].toUpperCase() + str.slice(1);
This snippet capitalizes the first letter of a string by isolating it, converting it to uppercase, and then appending the rest of the string. It's a simple and elegant way to ensure proper capitalization.
Example:
const str = "hello";
const capitalized = str[0].toUpperCase() + str.slice(1);
console.log(capitalized); // Outputs: "Hello"
10. Check if a String is a Palindrome
const isPalindrome = str => str === str.split('').reverse().join('');
This one-liner verifies whether a string is a palindrome by reversing it and comparing it to the original. If they are identical, the string is a palindrome.
Example:
const str = "racecar";
const isPalindrome = str => str === str.split('').reverse().join('');
console.log(isPalindrome(str)); // true
Conclusion
JavaScript one-liners are powerful tools that can make your code more elegant and efficient. By understanding and using these snippets, you can handle many common programming tasks quickly and with fewer lines of code. Experiment with these one-liners to find more ways to enhance your coding efficiency and readability.
Follow Us:
Stay updated with our latest tips and tutorials by subscribing to our YouTube Channel.